Here are the Top 12 Angular advanced interview questions. Let’s get started!
It is important to prepare well for an Angular developer interview. In later rounds the interviewer may ask Angular advanced interview questions preparing well will help demonstrate in-depth knowledge and experience with these advanced concepts can set a candidate apart from other Angular developers.
What is Easy and Lazy loading?
- Easy Loading : The easy loading technique is the standard loading methodology. Easy loading feature modules are loaded prior to the start of the program. It is frequently used in small-scale application.
- Lazy loading: The lazy loading technique dynamically loads feature module. This allows applications to run faster and is useful for larger projects where all modules are not required in the beginning.
Also read: 38 Smart Questions to Ask in a Job Interview
What is the view encapsulation in Angular?
View encapsulation in Angular can affect the component template and style of the entire program or vice versa. Three encapsulations techniques available are –
- Native: The component does not inherit styles from the main HTML. Styles defined in this component’s @Component decorator are only applicable to this component.
- Emulated (Default): The component inherits styles from the main HTML. Styles set in the @Component decorator are only applicable to this component.
- None: The component’s styles are propagated back to the main HTML and therefore accessible to all components on the page. Be wary of programs that have None and Native components. Styles will be repeated in all components with Native encapsulation if they have No encapsulation.
What is RxJs in Angular?
RxJS is an acronym meaning Reactive extensions for JavaScript. It is used to enable the use of observables in our JavaScript project, allowing us to do reactive programming. RxJS is utilized in many popular frameworks, including Angular since it allows us to compose our asynchronous or callback-based code into a sequence of operations executed on a data stream that releases values from a publisher to a subscriber. Other programming languages, such as Java and Python, offer packages that allow them to develop reactive programs utilizing observables.
Most of the time, rxJs is used in HTTP calls with angular. Because http streams are asynchronous data, we can subscribe to them and apply filters to them. This is one of the advanced interview questions asked several times in an interview.
Example: The following is a simple example of how RxJs can be utilized with HTTP calls.
let stream1 = httpc.get(“https://www.example.com/somedata”);
let stream2 = stream1.pipe(filter(x=>x>3));
stream2.subscribe(res=>this.Success(res),res=>this.Error(res))
Also read: Top 20 Angular version interview questions
Explain string interpolation and property binding in Angular.
- String interpolation and property binding are parts of data-binding in Angular.
- Data-binding is a feature in angular, which provides a way to communicate between the component(Model) and its view(HTML template).
- Data-binding can be done in two ways, one-way binding and two-way binding.
- In Angular, data from the component can be inserted inside the HTML template. In one-way binding, any changes in the component will directly reflect inside the HTML template but, vice-versa is not possible. Whereas, it is possible in two-way binding.
- String interpolation and property binding allow only one-way data binding.
- String interpolation uses the double curly braces {{ }} to display data from the component. Angular automatically runs the expression written inside the curly braces, for example, {{ 2 + 2 }} will be evaluated by Angular and the output 4, will be displayed inside the HTML template. Using property binding, we can bind the DOM properties of an HTML element to a component’s property. Property binding uses the square brackets [ ] syntax.
Explain the concept of Dependency injection.
Dependency injection is an application design pattern implemented in Angular. It forms one of the core concepts in Angular.
Dependency are services with functionality. Different components and directives can need functionality of a service. Angular framework provides a mechanism to add these dependencies to our components and directives. We are just making dependencies which are injectable across all components of an application. This is how Dependency Injectors (DI) works. Take the example of ng g service test
import { Injectable } from ‘@angular/core’;
@Injectable({
providedIn: ‘root’
})
export class TestService {
importantValue:number = 42;
constructor() { }
returnImportantValue(){
return this.importantValue;
}
}
As one can notice, we can create injectable dependencies by adding the @Injectable decorator to a class.
We inject the above dependency inside the following component:
import { TestService } from ‘./../test.service’;
import { Component, OnInit } from ‘@angular/core’;
@Component({
selector: ‘app-test’,
templateUrl: ‘./test.component.html’,
styleUrls: [‘./test.component.css’]
})
export class TestComponent implements OnInit {
value:number;
constructor(private testService:TestService) { }
ngOnInit() {
this.value = this.testService.returnImportantValue();
}
}
One can see we have imported our TestService at the top of the page. Then, we created an instance inside the constructor of the component and implemented the returnImportantValue function of the service.
From the above example, we can observe how angular provides a smooth way to inject dependencies in any component.
What is the difference between AOT and JIT?
AOT or Ahead Of Time compilation converts your code during the build time before the browser downloads the code. This ensures the browsers are render faster. To start AOT compilation, include the -aot option with the ng build and ng serve command.
JIT or Just-in-command compilation process is a way of compiling computer code to machine code during execution or run time. JIT is also known as dynamic compilation. JIT compilation is the default when you run the ng build and ng serve CLI command.
Also read: Top 15 Angular basic interview questions
What is dependency injection?
Dependency injection, or DI, is one of the fundamental concepts in Angular. DI is wired into the Angular framework and allows classes with Angular decorators, such as Components, Directives, Pipes, and Injectables, to configure dependencies that they need.
Two main roles exist in the DI system: dependency consumer and dependency provider.
How are component dependencies injected in a workflow?
The first step is to add the @Injectable decorator to show that the class can be injected.
@Injectable()
class HeroService {}
The next step is to make it available in the DI by providing it. A dependency can be provided in multiple places:
- At the Component level, using the providers field of the @Component decorator. In this case the HeroService becomes available to all instances of these component and other components and directives used in the template. For example:
@Injectable({
providedIn: ‘root’
})
class HeroService {}
When you provide the service at the root level, Angular creates a single, shared instance of the HeroService and injects it into any class that asks for it. Registering the provider in the @Injectable metadata also allows Angular to optimize an app by removing the service from the compiled application if it isn’t used, a process known as tree-shaking.
What are promises and Observables in Angular?
Promises and Observable both deal with Asynchronous events in Angular. Promises handles one event at a time and observables handle a sequence of events over time.
Promises give one value at a time. They execute immediately after creation and are not cancellable. They are Push errors to child promises.
Observables are executed only when subscribed to them using the Subscribe () method. They give multiple values over a period of time. They are used for functions like forEach, filter, and retry. Observable deliver errors to the subscribers. If you use the unsubscribe method() the listener stops receiving further values.
Also read: 8 Secrets of a Winning Interview Dress Code
What are ngOnInit()? How are they defined?
ngOnInit is a lifecycle hook and a callback method that is run by Angular to indicate that a component has been created. It takes no parameters and returns a void type.
export class MyComponent implements OnInit {
constructor() { }
ngOnInit(): void {
//….
}
}
What is bootstrap? How is it embedded in Angular?
Bootstrap is a strong toolkit. It is a collection of HTML, CSS, and JavaScript tools, developers use to build responsive web pages and applications. There are two ways to embed bootstrap library into your application.
- Angular BootStrap via CDN – Bootstrap CDN is a public Content Delivery Network. You can use it to load CSS and JavaScript remotely from its servers.
- Angular Bootstrap via NPM – Alternatively, you can add Bootstrap to your Angular project by installing it into your project folder by using NPM (Node Package Manager).
11.What is subscribing?
In Angular .subscribe() is a method of observable type. The observable type is a utility that have synchronous and asynchronous stream data to a variety of components or services that have subscribed to the observable. The subscribe method takes 3 methods as parameters-
- next: For each item being emitted by the observable perform this function
- error: If somewhere in the stream an error is found, do this method
- complete: Once all items are complete from the stream, do this method
For using cookies in Angular, you need to include a module called ngCookies angular-cookies.js.
To set Cookies – For setting the cookies in a key-value format ‘put’ method is used.
cookie.set(‘nameOfCookie’,”cookieValue”);
To get Cookies – For retrieving the cookies ‘get’ method is used.
cookie.get(‘nameOfCookie’);
To clear Cookies – For removing cookies ‘remove’ method is used.
cookie.delete(‘nameOfCookie’);
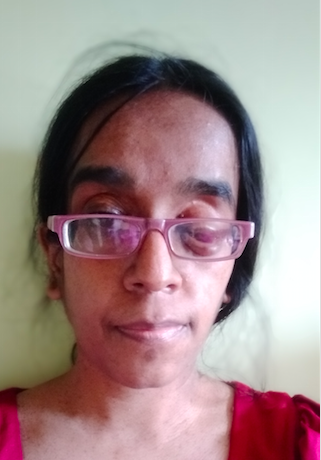
Shubha writes blogs, articles, off-page content, Google reviews, marketing email, press release, website content based on the keywords. She has written articles on tourism, horoscopes, medical conditions and procedures, SEO and digital marketing, graphic design, and technical articles. Shubha is a skilled researcher and can write plagiarism free articles with a high Grammarly score.
Leave a Reply