This article will discuss how to use Spotify API, Let’s get started!
Overview of Spotify Web API
Spotify is one of the leading digital music streaming platforms today. You get immediate access to its huge online music and podcast library, allowing you to listen to your favorite content whenever you choose. Spotify is a library that contains music from different genres and artists. There are podcasts on various topics.
Developers can use Spotify Web API to develop software that can communicate with Spotify’s streaming platform to retrieve content metadata, receive suggestions, make and manage playlists, or manage playback. In this blog, we discuss how to use the Spotify API.
How to use Spotify API – step-by-step integration guide
API is an application programming interface that is a server that you can connect to so that you transmit and receive data. Through a Web API, Spotify gives access to some of its user, playlist, and artist data to software and app developers.
Although Spotify’s web API is designed to support app integration and can be used for data analysis. In this section, we will focus on how to use Spotify API. We assume you have a premium or free Spotify API account. We will use curl to make API calls.
Step 1: Setup your account – to set up your account, you need to log in to the Spotify Developers Dashboard. Read and accept the terms and conditions to complete the setup.
Step 2: Create an app – any of the authorization flows can be used by an app to implement the Client ID and Client Secret required to submit a request for an access token. To create an App, go to your dashboard and click on “Create an App” and fill out the below required information.
- App Name: My_App
- App Description: This is my first Spotify app
- Redirect URI: You won’t need this parameter in this example, so let’s use http://localhost:3000.
Finally, check the Developer Terms of Service checkbox and tap on the Create button.
Step 3: Request an access token – the access token is a string that contains the credentials and permissions that can be used to access a given resource (e.g. artists, albums, or tracks) or user’s data (e.g. your profile or your playlists).
In order to request the access token you need to get your Client_ID and Client Secret:
- Go to Dashboards
- Click on the name of the app you just create My_app
- Click on the Setting button. Here you can find the Client ID and Client Secret and be found behind the “View Secret Link”.
Now that you have the credentials in hand you can access tokens.
- Send a POST request to the token endpoint URI.
- Add the Content-Type header set to the application/x-www-form-urlencode
- Add an HTTP body containing the Client ID and Client Secret, along with the grant_type parameter set to client_credentials.
curl -X POST “https://accounts.spotify.com/api/token” \
-H “Content-Type: application/x-www-form-urlencoded” \
-d “grant_type=client_credentials&client_id=your-client-id&client_secret=your-client-secret”
The response will return an access token valid for 1 hour
{
“access_token”: “BQDBKJ5eo5jxbtpWjVOj7ryS84khybFpP_lTqzV7uV-T_m0cTfwvdn5BnBSKPxKgEb11”,
“token_type”: “Bearer”,
“expires_in”: 3600
}
Step 4: Request artist data – in this example, we use the Get Artist endpoint to request information about the artist. According to the API Reference, the endpoint requires the Spotify_ID of the artist.
You can get the Spotify ID of an artist via the Spotify Desktop App”
- Search the Artist.
- Click on the three dots icons from artist’s profile.
- Select share > copy link to the artist. The Spotify ID is the value, you see after the open.spotify.com/artist URI.
Our API call must include the access token we have just generated using the Authorization header as follows:
curl “https://api.spotify.com/v1/artists/4Z8W4fKeB5YxbusRsdQVPb” \
-H “Authorization: Bearer BQDBKJ5eo5jxbtpWjVOj7ryS84khybFpP_lTqzV7uV-T_m0cTfwvdn5BnBSKPxKgEb11”
If there are no errors you will get JSON response.
Spotify API documentation references
Some useful Spotify API documentation references are given below:
- The Spotify Developers Documentation helps to clarify key concepts, contains tutorials for important topics, step-by-step guides that cover practical tasks and use cases, and provides references to the API documentation.
- The Spotify Web API developer’s console allows you to try out API requests and learn about their behavior.
- The Spotify Web API enables the development of software that can communicate with Spotify’s streaming platform in order to retrieve content metadata, receive suggestions, make and manage playlists, or manage playback.
- The Spotify Web API Authentication Guide refers to the process of granting a user or application access permission to Spotify data and features. Spotify implements the OAuth2.0 authorization framework and Client credentials.
- The Spotify Web API SDKs are supported by most online browsers like Chrome, Firefox, Safari, and Microsoft Edge for desktop (macOS, Windows, and Linux) and mobile (iOS and Android).
- The Spotify Developer forums are forums where developers can ask questions about developer tools, share feedback, and connect with other developers who build Spotify-specific applications.
- The Spotify Web API terms of service outline the terms and conditions of using the Spotify API, including usage guidelines and restrictions.
Spotify API examples for implementation
Below section has a list of examples, along with the code snippets –
1. How to use Python Spotify API?
Below is a list of examples along with code snippets on how to use the Python Spotify API:
- Before you begin, you need to create a Spotify developer account and register your application to obtain API credentials.
- Install the Spotify API wrapper – Spotify API wrappers such as Spotify help developers use the Spotify Web API more easily. Spotify is a Python library that provides a straightforward interface to the Spotify Web API. You can install the library using pip.
- Authenticate your application – You need to authenticate your application to access the Spotify Web API. Spotify uses OAuth 2.0 for authentication. You will need to redirect the user to the Spotify Login Page so that they can authorize your app to access their information. After access has been granted, Spotify will direct users back to your application with an access token. Use the access token to send authorized requests to the API.
- Access the Spotify Web API – Now that your application is authenticated, you can use the Spotify API to access the Spotify music catalog, podcasts, and user data. You can access a range of data, including track, album, artist information, playlist, and user data.
2. How to use Spotify API iOS?
Here are the steps to get started with using the Spotify API in iOS:
- Create a Spotify developer account at https://developer.spotify.com/. After signing up, create a new app and obtain your client ID and client secret.
- Download the latest version of the iOS SDK from GitHub.
- Add dependencies – add Spotify iOS packages to your project. You can do this through Swift Package Manager (SPM) or by adding the SpotifyiOS.framework or SpotifyiOS.xcframework to your Xcode project directly. You also need to add info.plist to your redirect URI that you registered at My Applications. Make sure to add your redirect URI under URL types and URL Schemes, and set a URL identifier as well. Finally, add #import <SpotifyiOS/SpotifyiOS.h> to your source files to import necessary headers.
- Check if Spotify is active and the user has authenticated your application.
3. How to use Spotify Android API?
Developers can use the Spotify Android SDK to build music-related apps for Android devices. The library is responsible for authorizing your app and fetching the access used to send requests to the Spotify Web API. You need to create a Spotify Android API project so that you can authenticate with it.
Visit the developer dashboard and login with your Spotify account and obtain a client ID and secret. Install Spotify App on the device. Install the version from PlayStore and Google Play.
Download the Spotify Android SDK from GitHub. Create your App, and ensure your App with at least one Activity or service in which you can put your code to connect to Spotify. Unzip the App Remote SDK file and add it to the library you are importing into the module.
Import dependencies if any and set up your Spotify credentials. Remember to Authorize your application to avoid failure. You need to have built-in authorization.
4. How to use Spotify API React?
Start by creating Spotify App on the Spotify dashboard. Click on the green button and confirm the terms and click the Create button. You will be directed to the Spotify app overview which will contain the Client ID and client secret. Open the terminal and create a new React application using the command
npx create-react-app spotify-react
This creates a react application. With cd spotify-react && yarn start you jump into the projects directly and start the development server which then runs at http://localhost:3000 by default.
To use the API the user needs to be authenticated with their Spotify Account. You need to create a link that leads us to the Spotify Authentication page. You can use the Spotify API React authentication methods to make requests for data such as playlists and artist information using the API’s provided methods.
Use React’s state and lifecycle methods to manage and update the data returned from the API, and render it in your app’s components. Handle any errors that may occur during the authentication or data request process, and ensure your app follows Spotify’s API usage guidelines.
Also read : What Developers Need To Know About Intellectual Property Rights?
5. How to use Spotify API Swift?
- To get started go to the Spotify Developers Dashboard and create the app.
- Install the latest version of Spotify from the Apple App Store. Download the latest Spotify iOS SDK from the GitHub repository.
- To Set up the iOS APK you need to register your Client ID and download a copy of Spotify iOS SDK on an iOS device.
- Import SpotifyiOS.framework and configure Info.plist.
- Set -ObjC Linked Flag and add Bridging Header.
- Remember to Set up User Authorization and Set up Remote.
6. How to use Spotify Graphql API?
To use the Spotify GraphQL API, follow these steps:
- Select the Spotify and Google Knowledge Graph APIs – Open GraphQL Studio by visiting the repository on GitHub. A pre-built list of API schemas is arranged alphabetically in the schema browser. Select Graph Search and Spotify.
- Generate a Google API key and a Spotify bearer token. The Google API key is relatively straightforward: just register a new app in your Google Cloud Project dashboard and enable the Knowledge Graph API at console.cloud.google.com/apis (find the API by name using Google’s “Welcome to APIs & Services” search bar). The Spotify API, on the other hand, requires an OAuth token that lasts for 3600 seconds. With your Google key and Spotify token plugged into the configuration options for each API, you’ll be able to run live requests instantly in GraphQL Studio.
- Set up your API locally and in the cloud.
- You can now test your API in GraphQL.
7. How to use C# Spotify API?
To use C# Spotify API you need to follow the steps below:
- Create a Spotify developer account and register your application to get your client ID and client secret. You need this information to authenticate your request to the API.
- Install your preferred Spotify API-Net package. The package allows you to integrate easily and provides a wrapper class with Spotify Web API. You can customize behavior. The package is easy to configure.
- Authenticate your application using the client ID and client secret.
- Use the Spotify Web API class to make a request to the Spotify Web API class. The class offers all the Spotify Web API’s endpoint functions/
How to get the Spotify API key?
It is easy to get the Spotify API key by following the steps below:
- Log into the Spotify developer’s dashboard using your Spotify account.
- Click on the App button.
- Enter your app name and app description in the dialogue box and agree to the terms of service and click ‘Create’.
- You will be directed to the App overview page. The app overview page has various information like the number of users, app status, client ID, and client secret.
- It is critical you store the Client ID and Client Secret in your code or a secure place for later use.
You need the Client Secret to authorize web API and SDK calls.
Is Spotify API free?
Yes, Spotify APIs are free. Spotify’s music data, including metadata, playlists, and user information, are all accessible through this API. You can use the free version, such as rate caps and restricted access to particular features. You need paid subscription to use some features such as access to a user’s own playlists.
What can you do with Spotify API?
Developers can connect to Spotify servers and offer end users features and functionalities that are specific to Spotify by using the pre-generated source code or Spotify APIs. Users can access the Spotify catalog, explore song metadata, and edit playlists, through external sites that have incorporated the Spotify API. The wealth of information provided by the APIs is useful for media and performance-based websites.
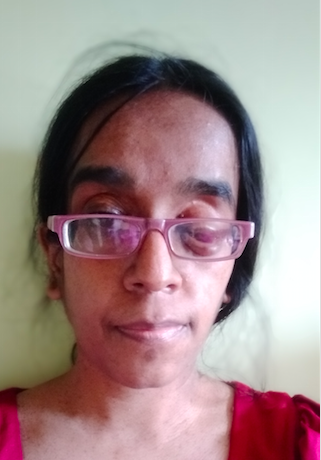
Shubha writes blogs, articles, off-page content, Google reviews, marketing email, press release, website content based on the keywords. She has written articles on tourism, horoscopes, medical conditions and procedures, SEO and digital marketing, graphic design, and technical articles. Shubha is a skilled researcher and can write plagiarism free articles with a high Grammarly score.
Leave a Reply