This article will discuss on how to use Slack API – a step-by-step integration guide and full documentation list. Let’s get started!
Overview of Slack API
Slack API grants provision to developers for creating custom applications, workflows, and data exchange both ways. The APIs provided by Slack are capable to query various types of information and accordingly create activities in a Slack-controlled workspace. You only need to choose the right Slack API, the details of which are available on the website.
List of Slack APIs and functionalities
As a first step towards how to use Slack API, let us first understand what are the different types of Slack integrations API and their functionalities.
- Slack Real Time Messaging API: It helps to display an event or a message inside the Slack instance or workspace in one or single feed. An instance can be, say suppose, you want to monitor when a member of a team reacts to a message. You can build a program using Slack API for real-time messaging.
- Slack Events API: It helps to control and specify a set of actions or events you want to monitor or capture in the Slack instance or workspace. For example, if you want to monitor a group of messages from a certain user, then it’s good to use this Slack API.
- Slack Web API: It works along with the Events API. While the Events API is responsible for sending information from the Slack instance to any other application, the Web API is responsible for receiving the response.
- Slack API conversations: It is just a subset of the Slack app API for Web, providing additional features of allowing changes to public, private, and shared channels and direct messages.
Also read: How to use ChatGPT API; a step-by-step integration guide and full documentation list
How to use Slack API – 7 step integration guide
Let us now discuss how to use Slack API, here are the steps.
- The Http RPC-style methods need to be called by passing on the following arguments
- GET querystring parameters
- POST parameters for example application/x-www-form-urlencoded
- It can also be a mix of both POST and GET parameters
- The methods accept arguments that are JSON attributes
- The files to be uploaded expect to send the parameters in the form of urlencoded
2. Set your content type header to application/x-www-form-urlencoded.
Here is the sample code.
POST /api/conversations.create
Content-type: application/x-www-form-urlencoded
token=xoxp-xxxxxxxxx-xxxx&name=something-urgent
And then present your key/value pairs
- In the HTTP header, transmit the bearer Slack API token. Here is an example of a code for displaying a message with certain menus.
POST /api/chat.postMessage
Content-type: application/json
Authorization: Bearer xoxp-xxxxxxxxx-xxxx
{“channel”:”C061EG9SL”,”text”:”I hope the tour went well, Mr. Wonka.”,”attachments”:[{“text”:”Who wins the lifetime supply of chocolate?”,”fallback”:”You could be telling the computer exactly what it can do with a lifetime supply of chocolate.”,”color”:”#3AA3E3″,”attachment_type”:”default”,”callback_id”:”select_simple_1234″,”actions”:[{“name”:”winners_list”,”text”:”Who should win?”,”type”:”select”,”data_source”:”users”}]}]}
- Next you need to authenticate the requests of Slack API. The step is achieved by providing the bearer token. To negotiate tokens or on how to get Slack API token, you need to register your application with Slack and obtain the required credentials. The token can be sent as an HTTP header or in the POST parameter.
- For returning results in Slack rest API, use the cursor-based approach. This will help to return a certain portion of the total results set or point to the next portion of the result set. A sample code for a request to the next page and limited to 2 results
GET slack.com/api/users.list?limit=2&cursor=dXNlcjpVMEc5V0ZYTlo%3D&token=xoxp-1234-5678-90123
- Limit your apps with 1 request per second for any API call in Slack API integration
- Use the event API to deliver events that count up to 30,000 deliveries per workspace per hour
- You can initiate your application via the Slack integration API in two ways – via a Slash command like using /mycallapp inside the composer. The other way is to use the Call icon.
- To set up the app you need to call the Slash command. The command buttons can be found under Add features and functionality. You will find the option to Create a new command.
- Slack then sends a payload to your app which looks as below,
{
“token”: “<verification token>”,
“team_id”: “T123ABC456”,
“team_domain”: “my-team”,
“channel_id”: “C123ABC456”,
“channel_name”: “test”,
“user_id” : “U123ABC456”,
“user_name”: “mattjones”,
“response_url”:”https://slack.com/callback/123xyz”,
“type”: “video”
}
- You then need to post your response to the response_url of the Slack connect API. You need to enter the following
{
“response_type”:”call”,
“call_initiation_url”:”https://join.call.com/123456″,
“desktop_protocol_call_initiation_url”: “call://join&room_id=123456”
}
- If you want to launch your app in desktop then use this desktop_protocol_call_initiation_url
- If you want the call type to be video then you need to ensure the initiation URL begins with a video. If its audo, then you need to enter the phone number received in the payload. Code sample
{
“response_type”: “audio”,
“call_initiation_url”: “https://your_company_url/…”,
“desktop_protocol_call_initiation_url”: “your_call_app_url://+1-202-555-0145”
}
- The calls.add method will now need to be used for adding the details of the call to the Slack webhook API. Store the id received as a response to refer while ending or updating the call
- Post the call to the channel. Sample code
“blocks”: [
{
“type”: “call”,
“call_id”: “R123”,
}
- Next you need to unfurl a call in the channel. The sample code is
{
“token”: “xxxx-xxxxxxxxx-xxxx”,
“channel”: “C123ABC456”,
“ts”: “12345.6789”,
“unfurls”: {
“https:\/\/url.to\/your\/call”: {
“blocks”: [{
“type”: “call”,
“call_id”: “Rxxx”
}]
}
}
}
- While responding to a rejected call, the following sample payload needs to be utilized
{
“token”: “12345FVmRUzNDOAuy4BiWh”,
“team_id”: “T123ABC456”,
“api_app_id”: “B123ABC456”,
“event”: {
“type”: “call_rejected”,
“call_id”: “RL731AVEF”,
“user_id”: “U123ABC456”,
“channel_id”: “D123ABC456”,
“external_unique_id”: “123-456-7890”
},
“type”: “event_callback”,
“event_id”: “Ev123ABC456”,
“event_time”: 1563448153,
“authed_users”: [“U123ABC456”]
}
- To update a call use calls.update_method and to and add or modify participants or users, use calls.participants.remove
- To end a call, use the calls.end method
- As a Slack API user, you can set up custom Slack API status, represented by the attributes status_text, status_emoji, and status_expiration profile attributes. You can retrieve the status of a user by using users.profile.get command
- You can set the custom status of a user through Slack API key or access token and entering into the users.profile.set method. The parameter for the profile must be a URL-encoded JSON string. The string must have the attribute as status_text
Sample code:
{
“status_text”: “riding a train”,
“status_emoji”: “:mountain_railway:”,
“status_expiration”: 0
}
- To unset the status, status_text, and status_emoji are set to empty
- You can expire the status of the user, using status_expiration command.
Sample code
{
“status_text”: “riding the train home”,
“status_emoji”: “:mountain_railway:”,
“status_expiration”: 1532627506
}
- To manually mark a user as auto or away, use the command users.setPresence
- For using slack bot API, use the command chat.postMessage for passing information
- Now coming to the usage of Slacks connect APIs that help users between organizations and different workspaces to work together in a Slack environment.
- You need to invite users from different organizations into the workspace, transforming the channel into a Slack Connect channel
- Once the app is installed in the target workspace, then you need to accept the invitations from the Slack Connect channel
- You can either approve, decline the invitations and then disconnect from the channel
- The other API which is of importance is the Slack Analytics API. You need to integrate within the channel enabling it to work along for providing insights and analysis of the way the company is leveraging Slack. Once it is integrated, it can extract the chats, messages, and members of the Slack API workspace.
Also read: How To Use YouTube API; A Step-By-Step Guide To Integrate And Full Documentation List
Slack API documentation references
Here are the key Slack API documentation references that serve as Slack API reference and Slack API tutorial.
- For overview of Slack API, refer here.
- To understand the connection protocols, and socket mode implementation, follow the Slack API docs.
- Now coming to the usage guides, if you want to learn about using the web API, then click here.
- To know about Calls API, refer to this link.
- Details on Bookmark API can be checked here.
- Now comes Slack connect and its APIs. Know more about it in the document link.
- The status API is another important Slack API which helps to check the health of the product. Here is the tutorial.
- For information on Slack API web methods, refer to this link,
- Then comes event types, the details of which can be checked here.
- And then the last, to check object types here is the link.
Slack API examples
Now let us see some Slack API examples, which will help beginners to be familiar with the coding, syntax, etc. These Slack API examples will further help to fast track the development activities, requiring considerably less time for completion. In the subsequent sections, we will be describing the same.
How to use Slack API Python
The Slack API Python SDK offers the full package for using the platform to install and build APIs. There are packages for Web API, Socket Mode, Webhooks, OAuth, and other APIs. Sample codes for real time API Slack python to refer for installation. Here is the reference.
The source code:
git clone https://github.com/slackapi/python-slack-sdk.git
cd python-slack-sdk
python3 -m venv .venv
source .venv/bin/activate
pip install -U pip
pip install -e .
Saving into test.py, a Slack real time messaging API example
# test.py
import sys
# Enable debug logging
import logging
logging.basicConfig(level=logging.DEBUG)
# Verify it works
from slack_sdk import WebClient
client = WebClient()
api_response = client.api_test()
How to use golang Slack API
As the first step towards how to use golang Slack API, you need to create .env file. Here is a sample code for Slack Golang API.
package main
import (
“fmt”
“os”
“time”
“github.com/joho/godotenv”
“github.com/slack-go/slack”
)
func main() {
godotenv.Load(“.env”)
token := os.Getenv(“SLACK_AUTH_TOKEN”)
channelID := os.Getenv(“SLACK_CHANNEL_ID”)
client := slack.New(token, slack.OptionDebug(true))
attachment := slack.Attachment{
Pretext: “Super Bot Message”,
Text: “some text”,
Color: “4af030”,
Fields: []slack.AttachmentField{
{
Title: “Date”,
Value: time.Now().String(),
},
},
}
_, timestamp, err := client.PostMessage(
channelID,
slack.MsgOptionAttachments(attachment),
)
if err != nil {
panic(err)
}
fmt.Printf(“Message sent at %s”, timestamp)
}
Here is the reference website on how to use Slack go API.
How to use Slack API PHP
In order to understand how to use Slack API PHP, you need build a Slack bot and API in php.
A sample code for creating a PHP application is elaborated below,
<?php |
/** |
* A lightweight example script for demonstrating how to |
* work with the Slack API. |
*/ |
// Include our Slack interface classes |
require_once ‘slack-interface/class-slack.php’; |
require_once ‘slack-interface/class-slack-access.php’; |
require_once ‘slack-interface/class-slack-api-exception.php’; |
use Slack_Interface\Slack; |
use Slack_Interface\Slack_API_Exception; |
// |
// HELPER FUNCTIONS |
// |
/** |
* Initializes the Slack object. |
* |
* @return Slack The Slack interface object |
*/ |
function initialize_slack_interface() { |
return null; |
} |
/** |
* Executes an application action (e.g. ‘send_notification’). |
* |
* @param Slack $slack The Slack interface object |
* @param string $action The id of the action to execute |
* |
* @return string A result message to show to the user |
*/ |
function do_action( $slack, $action ) { |
$result_message = ”; |
switch ( $action ) { |
default: |
break; |
} |
return $result_message; |
} |
// |
// MAIN FUNCTIONALITY |
// |
// Setup the Slack interface |
$slack = initialize_slack_interface(); |
// If an action was passed, execute it before rendering the page |
$result_message = ”; |
if ( isset( $_REQUEST[‘action’] ) ) { |
$action = $_REQUEST[‘action’]; |
$result_message = do_action( $slack, $action ); |
} |
// |
// PAGE LAYOUT |
// |
?> |
<html> |
<head> |
<title>Slack Integration Example</title> |
<style> |
body { |
font-family: Helvetica, sans-serif; |
padding: 20px; |
} |
.notification { |
padding: 20px; |
background-color: #fafad2; |
} |
input { |
padding: 10px; |
font-size: 1.2em; |
width: 100%; |
} |
</style> |
</head> |
<body> |
<h1>Slack Integration Example</h1> |
<?php if ( $result_message ) : ?> |
<p class=”notice”> |
<?php echo $result_message; ?> |
</p> |
<?php endif; ?> |
<form action=”” method=”post”> |
<input type=”hidden” name=”action” value=”send_notification”/> |
<p> |
<input type=”text” name=”text” placeholder=”Type your notification here and press enter to send.” /> |
</p> |
</form> |
</body> |
</html> |
How to use Slack API React
Before detailing how to use Slack API react, it is crucial to understand that this method is used to add reaction or emoji to a message.
Here is a Slack API react example on its usage:
The argument that you need to pass to the code are token like xxxx-xxxxxxxxx-xxxx, channel like C1234567890, name like thumbsup, timestamp like 1234567890.123456.
To add base emoji with a particular skin color, here is a Slack API code example,
thumbsup::skin-tone-6 or wave::skin-tone-3
After this particular call via the code mentioned above, the reaction is saved. Then an event is broadcast through the two types of APIs – events and RTM.
Also read: How to use Spotify API; a step-by-step integration guide and full documentation list
Slack API pricing
Now coming to Slack API pricing, there are 4 plans which you can choose from. There is a free version with limited facilities like only 10 integrations only. The other Slack API plans are Pro and Business+ where the Slack legacy API costs INR 218 per month and INR 375.20 per month respectively. The last one is Enterprise Grid for which you need to contact sales team.
Most used Slack APIs
In this section we will focus on the most used Slack APIs. This will provide an idea and guidance on the usage of a Slack API, and the purpose behind the use. You can then program your codes appropriately for your newly installed application.
Slack discovery API
A Slack discovery API helps to connect the Slack Enterprise Grid to the organization’s enlisted partners for data loss prevention or third party e-discovery where their cloud softwares reside. This connection is used to export messages and files from slack and even act on messages, accessible to all the users.
Slack API lookup user by email
The method for Slack API lookup user by email is used to find an user with a particular email address. You need to enter the token and the email id as the arguments to the code and access the method using the code GET.
Slack API reply to thread
The Slack API reply to thread method is used to retrieve the replies to a thread or a conversation. You need to use the conversations.replies method to achieve the desired result. The way to access the method Slack API thread is GET.
Slack API add user to channel
The method for Slack API add user to channel is conversations.invite. If the user is a member to the channel then you can add the person, the arguments being passed are the channel id and the users. The code for accessing the method is POST.
Slack API get messages from channel
The Slack API get messages from channel methods are used to access first the history of a Slack conversation and then extract the details of a certain message. The methods are conversations.list API method, conversations.history and conversations.replies.
Slack API create channel
The Slack API create channel method is conversations.create which helps to create a channel be it private or public and initiate the conversations within the channel. The name of the channels can contain numbers, lowercase letters. Underscores, hyphens and must be limited to max of 80 characters.
Slack API mention user
The Slacp API mention user tag is used to call a particular user in a list in a particular message or a conversation. Slack API tag user is used to tag using the symbol ‘@’ and select from the list or enter the name manually.
Slack API delete message
The Slack API delete message method is chat.delete which helps to delete a message in a conversation. If you use the method using the user token then it can delete messages which only the user is authorized to do so. And if you use the bot token then the messages accessible to the bot can only be deleted.
Slack API read messages
The Slack API read messages is an utility provided to retrieve the messages in a conversation or in a channel. The conversations.read method is specifically used for the purpose, where a specific message or a group of messages can be extracted.
Slack API edit message
The Slack API edit message method is chat.update API method. You can update any non-ephemeral message using the Slack API update message in a particular conversation or in a channel be it private or public.
Slack API send direct message
The Slack API send direct message method is called chat.postMessage. By passing the channel name and the block of messages as arguments, you can send direct messages in a conversation channel. You can post an attachment as well using this method using the Slack API send message to specific user method.
Slack API list channels
The Slack API lists channel method is conversations.list which helps in returning a list of channel where conversations occur in a Slack workspace. The channels returned as a response depends on the ones where the token has the access and also depends on the directives mentioned under the type parameter.
Slack API mention channel
Similar to tagging and mentioning a particular user in a conversation, the Slack API mention channel method is using ‘@’. You need to select the name of the channel from the list or enter the name manually after the symbol.
Slack API private channel
If you want to access a group of private channels then the Slack API private channel method groups.list can be used. It helps to retrieve all the channels to which the token has access to. If you want to initiate a conversation within a private channel then you can use the conversations API.
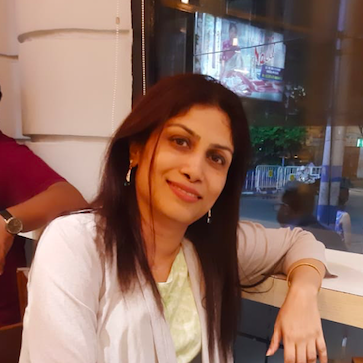
Kuntala is a versatile writer with a focus on diverse areas around work, productivity, collaboration at work, hiring, management, HR, and training. Her background of past experience in technology and consulting helps in molding razor-sharp insights into the research and user-focused content she creates. Professionally she is an IT consultant in a sales role and also a writer of short stories and poems, travel blogger, and fashion influencer.
Leave a Reply