This article will discuss about how to use Notion API with step-by-step integration guide and full documentation list. Let’s get started!
Overview of Notion API
Notion is a web-based productivity and note-taking application. It offers several organizational tools such as – task management, project tracking, to-do-lists, bookmarking, and much more. Developers and coders can use Notion public API to interact with databases, content, and pages within Notion. This is accomplished through a number of endpoints or locations where the API can interact with Notion and gather and arrange data from other sources (such as apps). Your team can create custom integrations or use ones that are already available.
Also read: How to use Instagram API; a step-by-step integration guide and full documentation list
Notion developer API and key functionalities
Developers use the Notion developer API to connect with other software to Notion or automate workflows. They can use the API to interact with Notion’s databases, pages, and content. This can be done through end-points- places where the API communicates and works with Notion and organizes information for your software. Here are some key features of Notion API:
- Create and update pages and databases
- Add and remove blocks
- Search for content
- Import and export data.
Developers can use Notion Integration API to build integration and automation. They can use Notion to connect to apps like Google Calendar, Gmail, and other productivity apps.
Some key functionalities of the Notion API are:
- For databases: information in Notion are stored in databases. Developers can use the Notion API component to pull a specific entry, search the database entry, and get a list of the entries in the database.
- For pages and blocks: Blocks are Notion pages that you can customize depending on your requirements. Developers can use the API to create a new page, set page properties, or retrieve page properties.
- For people in your workplace: you can use Notion to encourage transparency for all stakeholders in your organization. You can use Notion API to get names of all the users.
Developers can use these resources to learn more about the Notion Developer API. Here are a few great resources that you can use to learn more about the Notion Developer API:
- Notion Developer API documentation: The Notion Developer API documentation provides detailed information about the API’s endpoints, parameters, and responses.
- Notion Developer API examples: The Notion Developer API examples provide code samples that demonstrate how to use the API to perform common tasks.
- Notion Developer API community: The Notion Developer API community is a forum where you can ask questions and get help from other developers.
Also read: How to use Spotify API; a step-by-step integration guide and full documentation list
How to use Notion API – step-by-step integration guide
If you are familiar with coding you will not find it difficult to use Notion API. Before you begin you need to
- Sign up for a Notion account and create a new workspace or use an existing workspace.
- You need to have admin level access to the workspace.
- Create one or more database in the workspace.
- You need to install npm and node.js to use Notion’s JavaScript Library
Step 1: Create an integration
- Visit the website your browser.
- Click the + new integration button
- Name the integration
- Select the authorization for your integration.
- Click Submit button.
On the next page you can get notion API key or Notion integration token. You need to make a request to the Notion API.
Step 2: Share a database with your integration
After creating an integration you need to grant access to a database. To keep information secure, the integration should not have access specific pages. To share the database with your database
- Go to the database page in your workspace.
- Click the ••• on the top-right hand corner.
- Scroll to the bottom of the pop-up and click on Add connection
- Search for and select your integration of the Search for connection
Step 3: Save the database ID
You will need to save the database ID to manipulate the database using the integration. To get the database ID, simply copy your URL of database. The database ID is the string of characters after the /databases/ in the URL.
Once you have saved the database ID, you can start making requests to the Notion API.
Step 4: Make a request to the Notion API
To make a request to the Notion API, you will need to use the curl command-line tool. For example, to get all of the pages in a database, you would use the following command:
curl -X GET \
-H “Authorization: Bearer YOUR_API_KEY” \
Replace Your_API_Key with your Notion API key and Your_Database_ID with database you want to access.
Also read: How To Use YouTube API; A Step-By-Step Guide To Integrate And Full Documentation List
Notion API documentation references
The Notion API documentation is comprehensive and easy to navigate. The document contains detailed information about the API endpoints, parameters, and response. You can refer to the sample code to find out how to common task work.
The Notion API has following sections
- Introduction is important if you want to understand Notion API.
- Conventions The Notion API follows the RestFul API when possible. Requests and responses are encoded in JSON.
- Pagination: Endpoint that returns list of objects support cursor-based pagination requests.
- Response: The pages list the responses returned by each endpoint.
- Examples: This section contains code samples to show coders how to use the API for common tasks.
Here are some additional resources that you may find helpful:
- Notion Developer API community is a forum where you can ask questions and get help from other developers.
- Notion Developer API Blog provides news and development about the API.
- Notion Developer API Twitter account makes API announcements about new features.
Notion API examples
In this section we have covered how to use Notion API examples for different programming languages. These sections are a beginners guide on how to use the Notion API.
How to use Notion API Python
Start by setting up the Notion API and a database.
Follow all the steps mentioned in the previous section.
- Create an integration and get an API Key.
- Share your database with the integration.
- Save the database ID.
Set up the Python Code
To work with the API you will be working with the request module. You can install it with pip:
pip install request
Define your Notion API key, database ID in the header.
import requests
NOTION_TOKEN = “YOUR_INTEGRATION_TOKEN”
DATABASE_ID = “YOUR_DATABASE_ID”
headers = {
“Authorization”: “Bearer “ + NOTION_TOKEN,
“Content-Type”: “application/json”,
“Notion-Version”: “2022-06-28”,
}
Create pages in your Notion database
To create a new page, we send a POST request.
def create_page(data: dict):
create_url = “https://api.notion.com/v1/pages”
payload = {“parent”: {“database_id”: DATABASE_ID}, “properties”: data}
res = requests.post(create_url, headers=headers, json=payload)
# print(res.status_code)
return res
How to use Notion API NPM
Start by setting up the Notion API and database mentioned in the previous section. You will need the integration token or access token.
const { Client } = require(“@notionhq/client”)
// Initializing a client
const notion = new Client({
auth: process.env.NOTION_TOKEN,
})
Make a request to any Notion endpoint. You can get a complete list of endpoints from API reference.
;(async () => {
const listUsersResponse = await notion.users.list({})
})()
Each method returns a Promise which resolves the response.
console.log(listUsersResponse)
{
results: [
{
object: ‘user’,
id: ‘d40e767c-d7af-4b18-a86d-55c61f1e39a4’,
type: ‘person’,
person: {
email: ‘avo@example.org’,
},
name: ‘Avocado Lovelace’,
avatar_url: ‘https://secure.notion-static.com/e6a352a8-8381-44d0-a1dc-9ed80e62b53d.jpg’,
},
]
}
Endpoint parameters are grouped into a single object. You don’t need to remember which parameters go in the path, query, or body.
const myPage = await notion.databases.query({
database_id: “897e5a76-ae52-4b48-9fdf-e71f5945d1af”,
filter: {
property: “Landmark”,
rich_text: {
contains: “Bridge”,
},
},
})
How to use Notion API PHP
Start by setting up the Notion API and database mentioned in the previous section. To use the Notion API in PHP, you will need to install the Notion PHP package. You can do this using Composer:
composer require 64robots/php-notion
You need to create an instance of the Notion class using your Notion Internal Integration Token.
use R64\PhpNotion\Notion;
$notion = new Notion(‘secret_access_token’);
$database = $notion->databases()->retrieve(‘a65b5216-46cb-479b-961e-67cc7b05a56d’);
Testing
Composer test
How to use Notion SCIM API
- Your workspace must be on an Enterprise plan.
- Your Identity Provide (IdP) should support SAML 2.0 protocol.
- For a Notion workspace, only a Workspace Owner can configure SCIM.
Generate your SCIM API Token
Enterprise Plan Work workspace can generate and view SCIM API tokens by going to Settings & members → Security & identity → SCIM configuration.
- To generate a new token, click on the +New Token button in the right corner.
- A unique token is generated for each workspace owner.
Revoke tokens
When an owner leaves the workspace or their roles chance, their token is cancelled. The remaining Workspace owners will be informed to replace the revoked token when this occurs via an automated message delivered to all Workspace owners.
Additionally, every workplace owner in the workplace has the ability to revoke active tokens. Click next to the appropriate token to cancel it.
Replace existing tokens
Any integrations that currently use a token will require a replacement if it is revoked. Until it is replaced with an active token, any SCIM integration and user provisioning that depends on the revoked token will be disabled.
How to use Notion API JSON
JSON or JavaScript Object Notation is a lightweight format for storing and transporting data. It is used to when data is sent from the server to a web page. It is easy to understand.
To use Notion API you need to sign up and authenticate your Notion Account. You can follow the steps mentioned in the previous section.
Make a request
Now that you have your API key, you can make a request to the Notion API. The following code shows JSON data for a page.
const { Client } = require(‘@notionhq/client’);
const notion = new Client({ auth: process.env.NOTION_API_KEY });
(async () => {
const pageId = ’16d8004e5f6a42a6981151c22ddada12′;
const response = await notion.pages.get({ page_id: pageId });
console.log(response);
})();
This code will GET request to the /page/get endpoints. The page_id parameter is the ID of the page that you want to get data for.
The response object will contain the JSON data for the page. You can access the data by using the properties of the response object.
How to use Notion API JavaScript
Set up your development environment:
- Ensure you have Node.js installed on your system to run JavaScript code.
- Create a new directory for your project and navigate to it using the command line.
Initialize a new Node.js project:
- Run the command npm init in your project directory and follow the prompts to set up a new Node.js project. This will create a package.json file that manages your project dependencies.
- Now that you have the package.json file, install the packages you will be using for the project.
npm install –save @notionhq/client@^2.2.1
npm install –save dotenv@^16.0.3
The notionhq/client is the library that will help us use the Notion API easier and dotenv library makes it easier to use the secrets stored in the environment variables.
- Create an .env file to store the secret and database_id
NOTION_KEY=<Paste Your Internal Integration Token here>
NOTION_DATABASE_ID=<Pase your Notion Database ID here>
- You can start writing the JavaScript code in a file called index.js
const integrationToken = ‘YOUR_INTEGRATION_TOKEN’;
const headers = {
headers: {
Authorization: `Bearer ${integrationToken}`,
‘Content-Type’: ‘application/json’,
},
};
Now import the dotenv library and run the config method. Remember to load the variables stored inside the .env file into the environment. We are using console.log to print those variables to the screen to ensure they are working as expected.
You can now start interacting with the Notion API and database.
const Client = require(‘@notionhq/client’).Client
const dotenv = require(‘dotenv’)
dotenv.config()
const NOTION_CLIENT = new Client({ auth: process.env.NOTION_KEY })
const DATABASE_ID = process.env.NOTION_DATABASE_ID
async function getDatabaseData(client, databaseId) {
const response = await client.databases.query({
database_id: databaseId,
})
console.log(response)
}
getDatabaseData(NOTION_CLIENT, DATABASE_ID)
Here two constant variables are used throughout the file NOTION and DATABASE_ID.
How to use Notion API React
- We begin by creating a project directory and react app.
mkdir react-node-notion
cd react-node-notion
npx create-react-app@latest sample-app –template typescript
cd sample-app
npm run start
- Start with setting up Notion Integration mentioned in the previous section. Copy the secret integration token. Create your database and copy your database_id.
- Create an Express server. Notion provides a client library to make it easier to interact with the API from a backend server. Install these packages
npm install @notionhq/client cors body-parser dotenv
The CORS package allows the Express backend and React client to exchange data through the API endpoints. You can use the body-parser package to process incoming HTTP requests. You’ll parse the JSON payload from the client, retrieve specific data, and make that data available as an object in the req.body property. Lastly, the dotenv package makes it possible to load environment variables from a .env file in your application.
In the root directory of the server folder, create a .env file, and add the code below
NOTION_INTEGRATION_TOKEN = ‘your Integration secret token’
NOTION_DATABASE_ID = ‘database ID’
- Open the index.js file in the server project
const express = require(‘express’);
const {Client} = require(‘@notionhq/client’);
const cors = require(‘cors’);
const bodyParser = require(‘body-parser’);
const jsonParser = bodyParser.json();
const port = process.env.PORT || 8000;
require(‘dotenv’).config();
const app = express();
app.use(cors());
const authToken = process.env.NOTION_INTEGRATION_TOKEN;
const notionDbID = process.env.NOTION_DATABASE_ID;
const notion = new Client ({auth: authToken});
app.post(‘/NotionAPIPost’, jsonParser, async(req, res) => {
const {Fullname, CompanyRole, Location} = req.body;
try {
const response = await notion.pages.create({
parent: {
database_id: notionDbID,
},
properties: {
Fullname: {
title: [
{
text: {
content: Fullname
},
},
],
},
CompanyRole: {
rich_text: [
{
text: {
content: CompanyRole
},
},
],
},
Location: {
rich_text: [
{
text: {
content: Location
},
},
],
},
},
});
res.send(response);
console.log(“success”);
} catch (error) {
console.log(error);
}
});
app.get(‘/NotionAPIGet’, async(req, res) => {
try {
const response = await notion.databases.query({
database_id: notionDbID,
sorts: [
{
timestamp: ‘created_time’,
direction: ‘descending’,
},
]
});
res.send(response);
const {results} = response;
console.log(“success”);
} catch (error) {
console.log(error);
}
});
app.listen(port, () => {
console.log(‘server listening on port 8000!’);
});
- In the monitor
npm start
- Set up React Client
npm install axios
- Implement the POST and GET API Methods
Open the src/App.js file and replace the code
import React, { useState} from ‘react’;
import Axios from ‘axios’;
function App() {
const [name, setName] = useState(“”);
const [role, setRole] = useState(“”);
const [location, setLocation] = useState(“”);
const [APIData, setAPIData] = useState([]);
const handleSubmit = (e) => {
e.preventDefault();
Axios.post(‘http://localhost:8000/NotionAPIPost‘, {
Fullname: name,
CompanyRole:role,
Location:location
}).catch(error => {
console.log(error);
});
Axios.get(‘http://localhost:8000/NotionAPIGet‘)
.then(response => {
setAPIData(response.data.results);
console.log(response.data.results);
}).catch(error => {
console.log(error);
});
};
return (
<div className=”App”>
<header className=”App-header”>
<div className=”form”>
<form onSubmit={handleSubmit}>
<p>First Name</p>
<input
type=”text”
placeholder=”First name …”
onChange={(e) => {setName(e.target.value)}}
/>
<p>Company Role</p>
<input
type=”text”
placeholder = “Company Role….”
onChange={(e) => {setRole(e.target.value)}}
/>
<p> Company Role</p>
<input
type=”text”
placeholder = “Location….”
onChange={(e) => {setLocation(e.target.value)}}
/>
<button type=”submit”>Submit</button>
</form>
</div>
<div className=”Data”>
<p>API DATA</p>
{
APIData.map((data) => {
return (
<div key={data.id}>
<p>Name: {data.properties.Fullname.title[0].plain_text}</p>
<p>Role: {data.properties.CompanyRole.rich_text[0].plain_text}</p>
<p>Location: {data.properties.Location.rich_text[0].plain_text}</p>
</div>
)
})
}
</div>
</header>
</div>
);
}
export default App;
This section allows you to submit their name, role and user information.
- Start the React’s development server and go to local host on your browser to view results.
How to use Notion API iOS
- Start by creating Notion integration and create your Notion DB. Copy Notion API Key and database_ID
- Install Notion API for iOS. You can install it from the pod.
pod ‘NotionClient’, ‘0.0.1’
- Use following Notion API to fetch the database values
let client = NotionClient.init(token: “NOTION_INTEGRATION_SECRET_TOKEN“)client.queryDatabase(withId: “NOTION_DATABASE_ID“) { (data, error) inprint(data)}
- Replace the NOTION_INTEGRATION_SECRET_TOKEN with the API key and NOTION_DATABASE_ID by databse_ID.
Once you have replaced these values, you can run the code and it will fetch the database values from Notion
How to use Notion API Typescript
- Go up to Notion developer portal and create a new integration. The API key and secret key you’ll need to authenticate your requests will be sent to you as a result. You require a Notion database for storing and fetching data.
- Set up a new TypeScript project. You can use a project generator like Create React App or set up a project manually.
npx create-next-app blog_app –ts
The –ts flag allows your app to run using Typescript. This command will create a basic Next.js app inside the folder blog_app. Once the process is done, navigate to the blog_app folder using the command cd blog_app and install the notion client package:
npm install @notionhq/client
On the project root folder, create a .env file. This file will host the notion integration key that Next.js needs to access and connect with the notion API. Go ahead and add the two notion variables, the integration token key and the database id:
export NOTION_KEY=”your_notion_api_key”
export NOTION_DATABASE=”your_notion_database_id”
Replace your_notion_api_key with your actual Notion API Key and your_notion_database_id with your actual Notion database ID.
You can now access these environment variables in your code using process.env.NOTION_KEY and process.env.NOTION_DATABASE.
Start the development server to test the app
npm run dev
Go here; you should be able to view the default Next.js page.
How to use Flutter Notion API
- Set up a Notion integration using methods mentioned in the previous section.
- Install the Notion_API package
flutter pub get notion_api
- Import the Notion_API package
import ‘package:notion_api/notion_api.dart’;
- Create a new instance of the Client class and all the API requests will be available as a class properties method.
NotionClient notion = NotionClient(token: ‘YOUR SECRET TOKEN FROM INTEGRATIONS PAGE’);
- Use the NotionClient object to access the Notion API
final page = await notionClient.getPage(‘YOUR_PAGE_ID’);
How to use Golang Notion API
- Start by setting up your Notion integration and get the API key and database_id.
- Set up your new Go module. Open the terminal and navigate to your project directory to initialize a new Go module.
$ go get github.com/jomei/notionapi
- Create a new client object
import “github.com/jomei/notionapi”
client := notionapi.NewClient(“your-integration-token”)
- Use the client’s method to retrieve or update your content
page, err := client.Page.Get(context.Background(), “your-page-id”)
if err != nil {
// do something
}
- Handle errors if any
if (error != null) {
// Handle error
}
How to use Next.js Notion API
- Set up a Notion integration using methods mentioned in the previous section. This will give you the API key required to authenticate your requests.
- Set up a new Next.js project – you can use Next.js CLI or create a new project manually.
- Open a terminal and install required dependencies.
npm install notion-api-js
- In your Next.Js project create a file to handle API requests. Import the necessary modules and define functions to interact with the Notion API.
import { Client } from ‘@notionhq/client’;
// Create a new Notion client with your API key
const notion = new Client({ auth: process.env.NOTION_API_KEY });
// Fetch a Notion database by its ID
export async function fetchDatabase(databaseId) {
return notion.databases.retrieve({ database_id: databaseId });
}
// Fetch a Notion page by its ID
export async function fetchPage(pageId) {
return notion.pages.retrieve({ page_id: pageId });
}
Replace the database ID and API_key the actual ID and key from your Notion App.
- Start your server with
npm run dev
# or
yarn dev
You can verify the results in here.
Also read: How to use Shopify API; a step-by-step integration guide and full documentation list
Notion API pricing
Does Notion have an API? Yes, Notion does have an API. It is a Restful API that allows developers to interact with Notion’s database, pages, and content.
Is Notion free? Yes, developers can use the Notion API for commercial and personal projects. Notion does have paid models.
Free |
|
Plus (for small groups)
$8 per user/per month billed annually $10 billed monthly |
Everything in Free, and
|
Business (For companies using Notion to connect with several teams and tools)
$15 per user/per month billed annually $18 billed monthly |
Everything in Plus, and
|
Enterprise
(Advanced controls & support to run your entire organization.) |
Everything in Business, and
|
Also read: How to use Stripe API – a step-by-step integration guide and full documentation list
Most used Notion APIs
The most used Notion APIs are
- Notion Database API: The Notion database API is core how information is stored in Notion. You can use the API to interact with the Notion database, get specific database entries, update or delete entries, and get a list of all entries.
- Notion pages API: You can use the Notion page API to interact with pages. It can help create new page, set page properties or retrieve page properties.
- Notion block API: A block object represents a piece of content within Notion. The API translates the headings, toggles, paragraphs, lists, media, and more that you can interact with in the Notion UI as different block type objects.
- Notion Search API: The API searches all the parent and child pages that has been shared with the integration. It returns all the pages, blocks and database.
- Notion Integration API: The Notion integration API lets you connect Notion with other software.
Notion API create page
Notion API create page creates a new page that is a child of a existing page or a database. If the new page is a child of an existing page is the only valid property in the properties body param.
Notion API get page content
The Notion API get page content or working with page content allows you to read page content using the retrieve block children endpoint. The end point returns the list of children for any block that has children.
Notion API update page
The Notion API update page updates the properties page in the database. The properties body param of this end point can be used to update the properties of a page that is child of the database.
Notion API export
You can export a Notion page, database, or entire workspace in a variety of formats, including CSV, JSON, and Markdown. To export data, you can use the following steps:
- Click the ••• icon at the upper right corner of any Notion page.
- Choose Export from the dropdown menu.
- A window will pop up in the center of your screen. Select PDF from the dropdown menu.
- In the include content drop down, specify if you want to export everything or exclude files or images.
Notion API multi select
You can now dynamically create new options for select or multiple_select properties using the create_page and update_page endpoints.
Notion API table
The Notion API table has been added to the Notion API under Simple table. Tables are parent blocks for table row. They can contain only children of type table_row.
Notion API images
You can add external files and other media to the Notion using the API. Coders are responsible for the assets and making it available via a secured URL. Details of how to add files and media are available on the API.
Notion API filter
When you send query to the database, you can send a filter object in the body that limits the returned answers based on the specified criteria.
Notion API search
The Notion API search allows you to search the page and database shared in the integration. It returns all pages and databases, excluding duplicate linked database. You can search by title, content, and property values.
Notion API database id
The Notion API database id is a unique identifier that is assigned to each database in a Notion workspace. The database id can be used to make requests to the Notion API to access and manipulate data in the database.
Notion API relation
While creating or updating your database, you can now add relation and rollup property. The related database must also be shared with the integration.
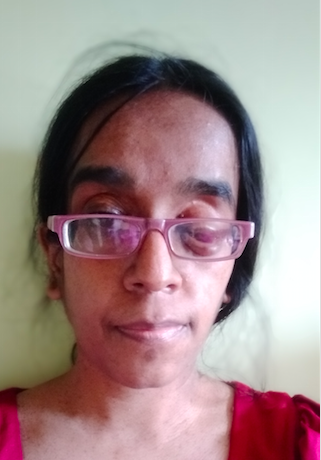
Shubha writes blogs, articles, off-page content, Google reviews, marketing email, press release, website content based on the keywords. She has written articles on tourism, horoscopes, medical conditions and procedures, SEO and digital marketing, graphic design, and technical articles. Shubha is a skilled researcher and can write plagiarism free articles with a high Grammarly score.
Leave a Reply