Here are Top 20 Angular version interview questions. Let’s get started!
Angular 2 interview questions
Angular2 interview questions and answers are useful to prepare well for an interview. Angular 2 interview questions can help demonstrate in-depth knowledge and experience with these advanced concepts can set a candidate apart from other Angular developers.
1.How is Dependency Hierarchy formed?
The dependency injection system of Angular is hierarchical. It consists of a tree of injectors that stands parallel to the application’s component tree. The Dependency Hierarchy is formed with the configurations. The reconfiguration of the injectors can be done at any given level of the component tree. The providers for different injectors can also be configured in the injector hierarchy.
2.What is the purpose of the async pipe?
The purpose of the async pipe is basically to mark the components that need to be checked for changes. It subscribes to an Observable or Promise and returns the latest value it has emitted. Once this new value gets emitted, the components are marked by the async pipe. Now, whenever any component is destroyed, the async pipe detaches or unsubscribes automatically. Similarly, if the expression reference of the component changes, the async pipe detaches or unsubscribes from the old Observable or Promise and subscribes to a new one.
Also read: 5 Ways to Stand Out in Your Next Job Interview
Angular 6 interview questions
Angular quiz helps you test your knowledge in Angular Framework. Here are Angular 6 interview questions are
1.Which is the following is correct about TypeScript?
- Angular is based on TypeScript.
- This is a superset of JavaScript.
- TypeScript is maintained by Microsoft.
- All of the above.
Answer Option d – All the above
2.What does AOT stand for?
- Ahead-Of-Time Compilation
- Angular Object Templates
- Both
- None of above
Answer – Option a- Ahead-of-Time compilation
Angular 4 interview questions
Angular 4 multiple choice questions are typically asked to gauge the concepts of the candidate. Here are some typical Angular 4 interview questions that may be asked during interview.
1.Router is part of which of the following module?
- @angular/core
- @angular/router
- Both
- None of the above
Answer – Option b-@angular/router
2.Which angular decorator allows us to define the pipe name that is globally available for use in any template in the across application?
- PipeName
- PipeDeco
- Pipe
- None of the above
Answer – Option c – Pipe
Angular 7 interview questions
Companies ask interview questions in Angular to find the best fit for their team. Angular 7 interview questions and answers for experienced will check their knowledge and ability to think outside-the-box.
1.What is parameterized pipe?
A pipe can accept any number of optional parameters to fine-tune its output. The parameterized pipe can be created by declaring the pipe name with a colon ( : ) and then the parameter value. If the pipe accepts multiple parameters, separate the values with colons. Let’s take a birthday example with a particular format(dd/MM/yyyy):
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-birthday’,
template: `<p>Birthday is {{ birthday | date:’dd/MM/yyyy’}}</p>` // 18/06/1987
})
export class BirthdayComponent {
birthday = new Date(1987, 6, 18);
}
Note: The parameter value can be any valid template expression, such as a string literal or a component property.
2.How do you chain pipes?
You can chain pipes together in potentially useful combinations as per the needs. Let’s take a birthday property which uses date pipe(along with parameter) and uppercase pipes as below
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-birthday’,
template: `<p>Birthday is {{ birthday | date:’fullDate’ | uppercase}} </p>` // THURSDAY, JUNE 18, 1987
})
export class BirthdayComponent {
birthday = new Date(1987, 6, 18);
}
Also read: Top 15 Angular basic interview questions
Angular 8 interview questions
Top Angular interview questions form an excellent resource to prepare for an interview, your Angular concepts for interview should be clear.
1.What is interpolation?
Interpolation is a special syntax that Angular converts into property binding. It’s a convenient alternative to property binding. It is represented by double curly braces({{}}). The text between the braces is often the name of a component property. Angular replaces that name with the string value of the corresponding component property.
Let’s take an example,
<h3>
{{title}}
<img src=”{{url}}” style=”height:30px“>
</h3>
In the example above, Angular evaluates the title and url properties and fills in the blanks, first displaying a bold application title and then a URL.
2.What are template statements?
A template statement responds to an event raised by a binding target such as an element, component, or directive. The template statements appear in quotes to the right of the = symbol like (event)=”statement”.
Let’s take an example of button click event’s statement
<button (click)=”editProfile()”>Edit Profile</button>
In the above expression, editProfile is a template statement. The below JavaScript syntax expressions are not allowed.
- new
- increment and decrement operators, ++ and —
- operator assignment, such as += and -=
- the bitwise operators | and &
- the template expression operators
Also read: Phone interview tips | How to ace a phone interview ?
Angular 9 interview questions
1.What is the difference between constructor and ngOnInit?
The Constructor is a default method of the class that is executed when the class is instantiated and ensures proper initialisation of fields in the class and its subclasses. Angular, or better Dependency Injector (DI), analyses the constructor parameters and when it creates a new instance by calling new MyClass() it tries to find providers that match the types of the constructor parameters, resolves them and passes them to the constructor.
ngOnInit is a life cycle hook called by Angular to indicate that Angular is done creating the component.
Mostly we use ngOnInit for all the initialization/declaration and avoid stuff to work in the constructor. The constructor should only be used to initialize class members but shouldn’t do actual “work”. So you should use constructor() to setup Dependency Injection and not much else. ngOnInit() is better place to “start” – it’s where/when components’ bindings are resolved.
export class App implements OnInit{
constructor(private myService: MyService){
//called first time before the ngOnInit()
}
ngOnInit(){
//called after the constructor and called after the first ngOnChanges()
//e.g. http call…
}
}
Angular 10 interview questions
1.What are components?
Components are the most basic UI building block of an Angular app, which form a tree of Angular components. These components are a subset of directives. Unlike directives, components always have a template, and only one component can be instantiated per element in a template. Let’s see a simple example of Angular component
import { Component } from ‘@angular/core’;
@Component ({
selector: ‘my-app’,
template: ` <div>
<h1>{{title}}</h1>
<div>Learn Angular6 with examples</div>
</div> `,
})
export class AppComponent {
title: string = ‘Welcome to Angular world’;
}
2. What is a service?
A service is used when a common functionality needs to be provided to various modules. Services allow for greater separation of concerns for your application and better modularity by allowing you to extract common functionality out of components.
Let’s create a repoService which can be used across components,
import { Injectable } from ‘@angular/core’;
import { Http } from ‘@angular/http’;
@Injectable({ // The Injectable decorator is required for dependency injection to work
// providedIn option registers the service with a specific NgModule
providedIn: ‘root’, // This declares the service with the root app (AppModule)
})
export class RepoService{
constructor(private http: Http){
}
fetchAll(){
return this.http.get(‘https://api.github.com/repositories’);
}
}
Also read: Amazing Tips to Standout for a Memorable Job Interview
Angular 11 interview questions
Angular Routing is a popular framework used for developing single-web applications. If you are applying for Angular routing, it is important to prepare Angular routing interview questions. We review Angular 11 interview questions.
1.What is Angular routing?
Angular routing is a way to manage the different states or views of an Angular application. It lets you define different URLs for different parts of your app, and map those URLs to the corresponding Angular components. Angular routing also lets you specify which components should be displayed when a user navigates to a specific URL.
2.Can you list the main features of Angular Router?
The Angular Router is a powerful routing library that allows developers to create single-page applications with rich routing capabilities. The router is easy to use and can be configured to work with a variety of different backends. The router also supports lazy loading, which can improve the performance of large applications.
Angular 12 interview questions
Angular 12 interview questions can help shortlist the best programmer for your firm. Here are some great Angular programming interview questions you can ask your candidates.
1.If your data model is updated outside the ‘Zone’, explain the process how will you the view?
You can update your view using any of the following:
- ApplicationRef.prototype.tick(): It will perform change detection on the complete component tree.
- NgZone.prototype.run(): It will perform the change detection on the entire component tree. Here, the run() under the hood will call the tick itself and then parameter will take the function before tick and executes it.
- ChangeDetectorRef.prototype.detectChanges(): It will launch the change detection on the current component and its children.
In HTML element can be easily hidden using the ng-hide directive in conjunction along with a controller to hide an HTML element on button click.
View
<div ng-controller=”MyController”>
<button ng-click=”hide()”>Hide element</button>
<p ng-hide=”isHide”>Hello World!</p>
</div>
Controller
controller: function() {
this.isHide = false;
this.hide = function(){
this.isHide = true; }; }
Angular 13 interview questions
Angular is a widely-used JavaScript single-page applications frameworks. It can be challenging to find Angular 13 interview questions. We have listed some Angular latest interview questions.
1.How does an Angular Application work?
The working of Angular is based on its components. The Angular Applications start working in the Angular.JSON configuration file. This file helps the builder locate the main file, configurations, and paths. Now the application starts.
Next comes the Main.TS file which serves as the entry point for the configuration file. It helps to create a browser environment to help application run.
The Angular application is now bootstrapped using the APP.MODULE.TS.. The app that component that gets bootstrapped is stored in the APP.COMPONENT.TS file. Now the INDEX.HTML file is called and is used to ask Angular to load the application component. After the component is loaded, the content gets displayed from the APP.COMPONENT.HTML file. This is how the Angular application works and the components discussed here help to achieve so.
2.What is metadata?
We instruct Angular on how to handle a class by using metadata. Unless we explicitly notify Angular that a component is being used, which we do with the aid of metadata, a component behaves as a class when it is used. A decorator is used in TypeScript to attach metadata. Decorators are functions that are familiar with the setup of classes and how they should operate.
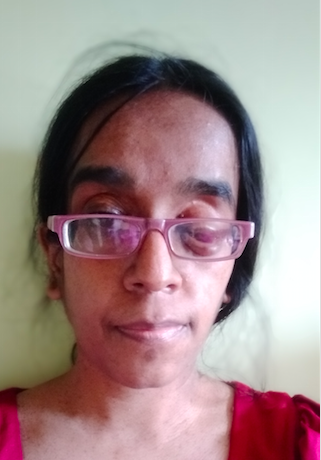
Shubha writes blogs, articles, off-page content, Google reviews, marketing email, press release, website content based on the keywords. She has written articles on tourism, horoscopes, medical conditions and procedures, SEO and digital marketing, graphic design, and technical articles. Shubha is a skilled researcher and can write plagiarism free articles with a high Grammarly score.
Leave a Reply