This article will discuss about how to use Airtable API with step-by-step integration guide and full documentation list. Let’s get started!
Overview of Airtable API
Airtable is a cloud-based platform for creating and managing databases. It is a spreadsheet-database hybrid with features of databases applied to a spreadsheet. Over 300,000 organizations use the platform because it helps teams create strong, adaptable apps on top of shared data. Airtable API is based on the Restful API. Programmers can build applications that can access and manipulate data on Airtable. It can be used to build custom integrations with other applications, automate workflows, and create custom reports and dashboards. The API is easy to use and supports a wide range of features, making it a powerful tool for developers and businesses.
Also read: How to use Stripe API; a step-by-step integration guide and full documentation list
Airtable developer API and key functionalities
You can use Airtable developer API to build integration into other applications or build stand-alone programs. You can access Airtable through the API to:
- Create, read, update, and delete records
- Create and manage views
- Create and manage formulas
- Create and manage automation
- Integrate with other applications
Some Airtable API integration scenarios are
- Create new records for platforms that are not supported.
- Update records for a third-party app.
- Update and delete records from a 3rd party platform.
How to use Airtable API – step-by-step integration guide
You must develop a backend application that serves as a proxy for the Airtable service in order to launch the Airtable REST API Integration. When customers want to communicate with Airtable via HTTP POST requests, the application must accept their requests. The following steps will add Airtable Rest api integration effortlessly:
Step1: Create an Airtable account and add email address
- Start by creating Airtable account. Click on Sign-up on the top left corner. Enter relevant information and click on Continue. You will need click the Activate My Account in your email.
- You can now create a username and password and click Save Changes.
- After signing up we recommend you click on Add email address so you receive notifications about updates Airtable account.
Step2: Click the new table
- Click Create Table. You will be redirected to a page where you can select the database you want to add your table to. Pick the option that best meets your needs. You can choose “Other” or “Custom” if you are unsure of the database type you want to add to your table.
- You will be prompted to name the table and description. You will then be sent to the New Row form. By clicking on each column heading in this area, you can add columns. Choose the desired columns, then enter the necessary data.
- Click on Save Changes button.
- The Airtable team will send you the approval via email.
Step3: Connect your table any Rest API
You need to connect your table to a Rest API.
- Click on the create connection link
- Select the API from the choose from the selection available.
Step4: Authorization
- Complete the authorization form in accordance with your requirements, then send the request. Verify that the request was delivered successfully. Obtain an authorization token, sign in to Airtable, and then modify it to suit your needs.
Also read: How to use ChatGPT API; a step-by-step integration guide and full documentation list
Airtable API documentation references and examples
The Airtable API documentation references is a comprehensive documentation for all end points, methods, and parameters. It provides everything you need to leverage Airtable’s powerful features. The Airtable API examples will help you understand how to use Airtable API.
You need to Login to the Airtable Account and create a base. Generate an API key for Airtable Integration.
How to use Airtable Python API
Here is how to use Airtable Python API
Step1: Install the airtable-request.py; $ pip install requests
Step2: Create an Airtable base and new table through the web interface.
import airtable
at = airtable.Airtable(‘BASE_ID’, ‘API_KEY’)
at.get(‘TABLE_NAME’)
Step3: Use API references to get the results.
An Airtable python api example to get table name and fetch one or multiple records
at.get(table_name, record_id=None, limit=0, offset=None,
filter_by_formula=None, view=None, max_records=0, fields=[])
Where
table_name (required) is a string representing the table name
record_id (optional) is a string, which fetches a specific item by id. If not specified, all items are fetched
limit (optional) is an integer, and it can only be specified if record_id is not present, and limits the number of items fetched (see pageSize in the AirTable documentation)
offset is a string representing the record id from which we start the offset
filter_by_formula (optional) is a string to filter the retrieving records (see filterByFormula in the AirTable documentation)
max_records (optional) is the total number of records that will be returned (see maxRecords in the AirTable documentation)
fields (optional) is a list of strings with the field names to be returned
How to use Airtable Javascript API
Here is how to use Airtable.js API
Step1: Install the Airtable.js in the node project; npm install airtable3.
Step2: To use the Airtable.js file you need use this link.
For demo you can run:
cd test/test_files
python -m SimpleHTTPServer
Edit the test/test_files/index.html and replace the BASE_ID and API_KEY with your ID.
Open this in your browser.
Step3: You can use the Airtable objects to interact with the database. Airtable javascript api example will explain more in detail.
const airtable = new Airtable(‘<YOUR_API_KEY>’);
const updatedRecord = {
“name”: “Jane Doe”,
“email”: “janedoe@example.com”
};
airtable.updateRecord(‘Your Base ID’, ‘Your Table ID’, 1234567890, updatedRecord);
How to use Airtable JSON API
Here is how to use Airtable database API JSON
Step1: Identify your base and table in step two
Choose the base and table within Airtable that you want to use the JSON API to interact with.
Step2: Construct the API endpoint URL
The Airtable JSON API’s root URL is https://api.airtable.com/v0/. To create the endpoint URL, add your base ID and table name to the base URL.
Step3: Make HTTP requests
Make HTTP calls to the created API endpoint URL using your favourite programming language or tool. You can send messages using libraries like Axios, Requests, or fetch.
Step4: Add the required headers
Include the required headers for content type and authentication in your API queries. Set the ‘Authorization: Bearer YOUR_API_KEY’ header in the Authorization section of the request. Additionally, change the Content-Type header to ‘application/json’.
Step5: Define the parameters and request method
Depending on the operation you want to carry out (such as retrieve records, create records, update records, or remove records), choose the appropriate HTTP method (GET, POST, PUT, remove). According to the API documentation, include any necessary arguments in the request URL or request body.
Step6: Handle the API response
Once you have the API response, process and handle it in accordance with the needs of your application. Parsing the JSON response, managing errors, and extracting the pertinent data may all be necessary. You can as
Step7: Develop your integration
As you need them, add more API functionalities, such as filtering, sorting, pagination, and attachments, to your integration.
How to use Airtable API NPM
Here is Airtable API node example
Start by initializing the project and install dependencies to start the server. You need to install the following :
- body-parser – a middleware to parse the body of incoming requests
- cors – ho handle cors headers
- express – to spin up our expressjs server
- morgan – a middleware utility tool that logs the server events (this is not essential but useful for debugging)
- node-fetch – fetch API for node environment
npm i express cors morgan body-parser node-fetch
After installing everything create app.js file
const express = require(“express”);
const app = express();
const cors = require(“cors”);
const bp = require(“body-parser”);
const fetch = require(“node-fetch”);
app.use(cors());
app.use(bp.urlencoded({ extended: false }));
app.use(bp.json());
app.use(require(“morgan”)(“dev”));
const port = process.env.PORT || 5000;
app.listen(port, () => {
console.log(“listning on ” + port);
});
Run this by node.app.js
To read the table we create an Airtable api nodejs server to view existing data in our table.
const express = require(“express”);
const app = express();
const cors = require(“cors”);
const bp = require(“body-parser”);
const fetch = require(“node-fetch”);
app.use(cors());
app.use(bp.urlencoded({ extended: false }));
app.use(bp.json());
app.use(require(“morgan”)(“dev”));
const AIRTABLEAPI = require(“./config/env”).airtableapikey; // import airtable api key
const AIRTABLEBASEID = require(“./config/env”).airtablebaseid;// import airtable base id
const AIRTABLETABLENAME = “seriescharacters”; // table name
const port = process.env.PORT || 5000;
app.get(“/view”, (req, res) => {
//we need to send a “GET” request with our base id table name and our API key to get the existing data on our table.
fetch(
`https://api.airtable.com/v0/${AIRTABLEBASEID}/${AIRTABLETABLENAME}?view=Grid%20view`,
{
headers: { Authorization: `Bearer ${AIRTABLEAPI}` } // API key
}
)
.then((res) => res.json())
.then((result) => {
console.log(result);
res.json(result);
})
.catch((err) => {
console.log(err);
});
})
How to use Airtable API to office 365
There are a few ways to use the Airtable API to Office 365. One way is use Airtable Zapier Integration.
- Start by creating an Zapier and Office 365 account.
- Go to the top left-hand corner of the Zappier account and press Zap.
- Name your Zap e.g. AirtableTOOffice
- From Zappier select Airtable.
- Choose event from the drop down menu and press Continue.
- Set up your trigger.
- Select Office 365 as the action app.
- Choose the action that you want to take.
- Connect your Airtable and Office 365 accounts.
- Test the Zap.
How to use Airtable API with WordPress
- Go to the Air Sync plugin page and click on Connections>> Add New.
- Enter a name for your new Connection.
- Fill in the Airtable Settings with the API Key.
- Select the form (article, page, etc.) in which you want to import the content from your table and configure the additional options.
- Link your link with fields in WordPress. Names in Airtable columns are case-sensitive, take care to adhere to the exact spelling.
- Select Strategy and Trigger from the Sync Settings menu.
- Once the connection is published, you’re done!
How to use Airtable jquery API
Requirement
- A server with PHP installed.
- cURL enabled
Here is how you can make a request to the Airtable ‘s API
$api_key = ‘YOUR_API_KEY’;
$base = ‘YOUR_SPREADSHEET_ID’;
$table = ‘YOUR_TABLE_NAME’;
$airtable_url = ‘https://api.airtable.com/v0/’ . $base . ‘/’ . $table;
$url = ‘https://api.airtable.com/v0/’ . $base . ‘/’ . $table . ‘?maxRecords=10&view=Main%20View’;
$headers = array(
‘Authorization: Bearer ‘ . $api_key
);
$ch = curl_init();
curl_setopt($ch, CURLOPT_HTTPGET, 1);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_TIMEOUT, 10);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_URL, $url);
$entries = curl_exec($ch);
curl_close($ch);
$airtable_response = json_decode($entries, TRUE);
- This code gets the data from your Airtable and converts into a PHP array with json_decode(). The output will be as follows
“records”: [
{
“id”: “recMGvRShuBoKakfY”,
“fields”: {
“grocery”: “bread”
},
“createdTime”: “2016-10-25T12:18:53.000Z”
}
],
“offset”: “recMGvRShuBoKakfY”
We convert the JSON to PHP array and use a for loop,
foreach($airtable_response[‘records’][‘fields’] as $key => $value) {
$string.= $value[‘Data’] . ‘, ‘;
$labels.= ‘”‘ . $value[‘Label’] . ‘”, ‘;
}
$data = trim($string, “,”);
$labels = trim($labels, “,”);
?>
How to use Airtable PHP API
Step1: Installation
If you are using Composer, you can run the following command.
composer require sleiman/airtable-php
Alternatively, directly download and extract them into your web directory.
Step2: Add Wrappers to your projects
If you are using Composer, run the autoloader
require ‘vendor/autoload.php’;
or include Airtable.php file
include(‘../src/Airtable.php’);
include(‘../src/Request.php’);
include(‘../src/Response.php’);
Step3: Initialize the class
use \TANIOS\Airtable\Airtable;
$airtable = new Airtable(array(
‘api_key’ => ‘API_KEY’,
‘base’ => ‘BASE_ID’
));
Step4: Get all the entries in the table
$request = $airtable->getContent( ‘Contacts’ );
do {
$response = $request->getResponse();
var_dump( $response[ ‘records’ ] );
}
while( $request = $response->next() );
print_r($request);
Also read: How To Use Notion API a step-by-step Integration Guide and Full Documentation List
How to use Airtable API in R
Developers can use some R packages, if they want to connect the R. In this example we wil use Darko Bergant’s package.
- Install devtools, you need to run
install.packages(“devtools”).
- Install Darko Bergant’s package.
devtools::install_github(“bergant/airtabler”)
- Now that you have installed devtools and bergant/airtabler installed you need load them into the session to be used. First
library(airtabler), then library(dplyr).
- To retrieve the data as a data frame you will need the API key.
Sys.setenv(“AIRTABLE_API_KEY”=”<Your API key”) #example key**************
- You also need to add the base that you want to pull the data from
airtable <- airtabler::airtable(“<base key>”, “<Tab/sheet name>”) #base key can be found in the API docs
- Now that we have the data.frame we can use the dplyr function
sample_n(airtable, 1) #1 is the number of rows we want
This is our result.
ID : 17 re*************
Twitter Handle : @amymcdougall96
Win a T Shirt : Yes
Name : Amy McDougall
Created Time : 2018-05-18T13:38:58.000Z
How to use Airtable C# API
Airtable.net is the C# sharp client of the public APIs of Airtable. . It creates a Windows class library called AirtableClientApi.dll that is intended for.NET Standard. By eliminating the need to worry about interacting with raw HTTP, low-level ideas like HTTP status codes, and records paging, AirtableClientApi.dll makes it easier to use Airtable APIs. By utilising programmatically available Airtable public APIs including List Records, Create Record, Retrieve Record, Update Record, Replace Record, and Delete Record, users can create C-Sharp apps that interface with Airtable.
Install the latest nuget package Airtable.1.3.0.nupkg.
Download the c# source code for Airtable.net at github.com. Simply add these source files to a new C#.NET Standard Class Library project in Visual Studio to compile the project to an assembly.
using System;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using System.Linq;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Text.Json;
using System.Text.Json.Serialization;
using AirtableApiClient;
readonly string baseId = YOUR_BASE_ID;
readonly string appKey = YOUR_APP_KEY_OR_ACCESS_TOKEN;
string offset = null;
string errorMessage = null;
var records = new List<AirtableRecord>();
using (AirtableBase airtableBase = new AirtableBase(appKeyOrAccessToken, baseId))
{
//
// Use ‘offset’ and ‘pageSize’ to specify the records that you want
// to retrieve.
// Only use a ‘do while’ loop if you want to get multiple pages
// of records.
//
do
{
Task<AirtableListRecordsResponse> task = airtableBase.ListRecords(
YOUR_TABLE_ID_OR_NAME,
offset,
fieldsArray,
filterByFormula,
maxRecords,
pageSize,
sort,
view,
cellFormat,
timeZone,
userLocale,
returnFieldsByFieldId,
includeCommentCount);
AirtableListRecordsResponse response = await task;
if (response.Success)
{
records.AddRange(response.Records.ToList());
offset = response.Offset;
}
else if (response.AirtableApiError is AirtableApiException)
{
errorMessage = response.AirtableApiError.ErrorMessage;
if (response.AirtableApiError is AirtableInvalidRequestException)
{
errorMessage += “\nDetailed error message: “;
errorMessage += response.AirtableApiError.DetailedErrorMessage;
}
break;
}
else
{
errorMessage = “Unknown error”;
break;
}
} while (offset != null);
}
if (!string.IsNullOrEmpty(errorMessage))
{
// Error reporting
}
else
{
// Do something with the retrieved ‘records’ and the ‘offset’
// for the next page of the record list.
}
- How to use Airtable API React
- How to use Airtable API Java
How to use Airtable Ruby API
Airtable has an alternate library called Airrecord
Step1: You can line to your Gemfile; gem ‘airrecord’
Step2: You can create an Airtable object and use methods to modify the code
Airrecord.api_key = “key1”
class Tea < Airrecord::Table
self.base_key = “app1”
self.table_name = “Teas”
has_many :brews, class: “Brew”, column: “Brews”
def self.chinese
all(filter: ‘{Country} = “China”‘)
end
def self.cheapest_and_best
all(sort: { “Rating” => “desc”, “Price” => “asc” })
end
def location
[self[“Village”], self[“Country”], self[“Region”]].compact.join(“, “)
end
def green?
self[“Type”] == “Green”
end
end
class Brew < Airrecord::Table
self.base_key = “app1”
self.table_name = “Brews”
belongs_to :tea, class: “Tea”, column: “Tea”
def self.hot
all(filter: “{Temperature} > 90”)
end
def done_brewing?
Time.parse(self[“Created At”]) + self[“Duration”] > Time.now
end
end
teas = Tea.all
tea = teas.first
tea[“Country”] # access atribute
tea.location # instance methods
tea.brews # associated brews
How to use Airtable API Express Js
- Install the Airtable.js library.
npm install airtable
- Create a Express JS application
const express = require(‘express’);
const app = express();
- Import the Airtable.js library into your application.
const airtable = require(‘airtable’);
- Create an Airtable API client and make a request
const client = new airtable.Base(‘YOUR_API_KEY’, ‘YOUR_BASE_ID’);
const records = await client.getRecords(‘Table Name’);
- Handle response
records.forEach(record => {
// Do something with the record.
});
Also read: How to use Slack API; a step-by-step integration guide and full documentation list
Airtable API pricing
Airtable has different pricing models for its API. Airtable API pricing model is as follows:
Free | Plus | Pro | Enterprise |
($10 per seat/per month) billed annually $12 billed monthly | ($20 per seat/per month) billed annually $24 billed monthly | For departments and organizations who need a secure, scalable, and customizable connected apps platform to stay aligned and move work forward, contact Airtable Sales department. | |
|
|
|
|
Most used Airtable
Airtable is a powerful platform that allows you to create and manage data flexibly and collaboratively. You can build some powerful apps and automate workflows using Airtable for data storage and management. In this section, we focus on the most used Airtable APIs.
Airtable scripting API
The Airtable scripting API is an API reference for scripting that lets you create short scripts using scripting to save time on monotonous operations and gain deeper insights using advanced queries and bespoke reports.
Airtable API create table
The Airtable API create table lets you create a new table and return the schema for the table. The table contains columns that define the data structure, you can specify the column type, names, and other properties.
Airtable API update record
The Airtable API update record lets you update a single record with the table name or tableID. The body of our request should contain an array of up to 10 record objects. Each of these objects should have a field property containing all of your record’s cell values by field name or a field id for all of your record’s cell values by field name, as well as an id property that represents the record ID.
Airtable API upload attachment
The Airtable API upload attachment lets you upload an attachment. However, the attachment must exist in a public URL you can use. Start by uploading your file on a web service and get a URL. You can use the URL for your create/update requests.
Airtable API filter
There are two ways the Airtable API filter works.
- Passing a view parameter in an API call with the list records scope can filter records by only returning those that appear in a certain table view.
- The filterByFormula parameter is another method for filtering records in a call with a list records scope.
Airtable get data from API
You can use Fetch API, your script can send network queries to outside services.
async function (url: string | Request, init?: object) => Promise<Response>;
Airtable metadata API
You can use Airtable metadata API to get details about Airtable data, such as a table’s schema or a list of all the tables in a base, using the. This data can be used to automate operations or combine Airtable with other programs.
Airtable API attachments
The attachment field is a field type of the field that allows you to attach one or more files to your Airtable account.
Airtable standard API
The Airtable Standard API or web API
- Airtable API get linked records
- Airtable store API
- Airtable API offset
Airtable sync API
The Airtable sync API allows you to bring information from external applications into a single, centralized table within the App. The API is available to Pro or Enterprise users only.
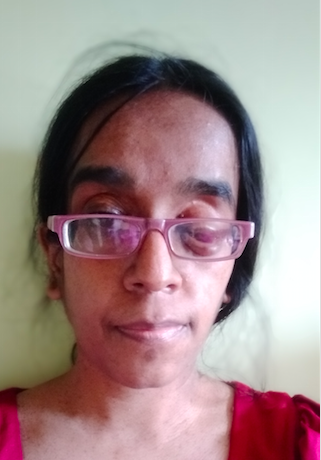
Shubha writes blogs, articles, off-page content, Google reviews, marketing email, press release, website content based on the keywords. She has written articles on tourism, horoscopes, medical conditions and procedures, SEO and digital marketing, graphic design, and technical articles. Shubha is a skilled researcher and can write plagiarism free articles with a high Grammarly score.
Leave a Reply