This article will discuss about how to use Yahoo Finance API with step-by-step integration guide and full documentation list. Let’s get started!
Overview of Yahoo Finance API
Many of us are aware of Yahoo Finance but then there are questions on Does Yahoo Finance have an API? Yes, it does. Yahoo Finance API contains several methods/ APIs/libraries to obtain real-time and historical data for financial market offerings and products.
Yahoo finance developer API and key functionalities
Yahoo Finance Developer API provides several functions and features. The key ones are
- Accessing financial data through Yahoo API Finance, like mutual funds, futures, stocks, and other securities
- Build custom applications and analytical tools using the financial data of Yahoo Finance API, for example, pricing alerts, stock portfolios
- Access comprehensive financial information like historical quotes and news from the stock market
- Yahoo Finance API commercial use includes monitoring portfolios, analysis of the stock market, and create trading systems and interactive charts and tools
- Yahoo Finance API fields can capture data for performing high-end financial activities like investment strategies, monitoring the portfolio, and trends in the stock market
- Another important functionality of Yahoo Finance API is its access to currency exchange rates and market indices
- It is free to use for personal objects but for commercial usage, it needs a paid subscription
- Yahoo Finance API helps to access information on an analyst’s recommendations about the company
- It provides information about the company’s earnings, top shareholders of a stock, and the company’s earnings trend
- The data retrieved by the API can be exported into spreadsheets for further deep dive analysis
Also read: How to use ChatGPT API – a step-by-step integration guide and full documentation list
How to use Yahoo Finance API – step-by-step integration guide
Let us start to be acquainted with how to use Yahoo Finance API.
-
The first point to be discussed is the OAuth protocol for authorization and authentication. Yahoo Finance Public API supports two primary profiles – server-side application and client-side application.
1.1 The initial step is to receive the Yahoo network credentials
1.2 Next is to obtain the authorization Yahoo Finance API URL
1.3 The third is to provide the authorization to access the data
1.4 And finally need to get the access token and refresh token of the Yahoo Finance API key
-
The second important point is using Yahoo Finance API for Analytics and reporting.
2.1 The sample request for receiving data on the sessions
2.2 The sample request for obtaining data about an event
2.3 The sample for adding Yahoo Finance API parameters to an event
2.4 The sample request for obtaining real time data
2.5 The sample request for obtaining revenue analytics data
-
Thirdly, let’s discuss how to use Yahoo Finance Restful API – Monetization Reporting API. You need to interact with the API through HTTPS Get requests. You need to acquire a Yahoo Finance Rest API token.
3.1 If you want to query the monetization data, then you need to select the appropriate Yahoo Finance Data API requests from here
3.2 To aggregate the metrics, you need to use time grain and dimension
3.3 If you want to provide a date/time range then use the dateTime
3.4 If you want to filter out the dimension values then use Filters
3.5 If you want to sort out the columns in metrics then the code is as below - If you want to leverage the Yahoo Finance API limits or rate limits, then you can restrict to a few 100 requests per day. If you want to increase the limit, then you need to go for an additional Yahoo Finance API license by subscribing to Market data plans or a proxy plan where you can increase the rate limit to 10,000 API calls in a day.
-
Next is to understand how to install the Yahoo_Fin library,
5.1 the command is pip install yahoo_fin
5.2 The command for installing requests_html is pip install requests_html
5.3 The library has two modules stock_info and options -
The method for retrieving the finance fundamental data like the Price to Earnings
An illustration of the results returned is below::quote_table = si.get_quote_table(“aapl”, dict_result=False)
quote_table
{‘1y Target Est’: 314.55,
’52 Week Range’: ‘174.52 – 327.85’,
‘Ask’: ‘323.97 x 1300’,
‘Avg. Volume’: 48563118.0,
‘Beta (5Y Monthly)’: 1.17,
‘Bid’: ‘322.30 x 1000’,
“Day’s Range”: ‘317.23 – 322.35’,
‘EPS (TTM)’: 12.73,
‘Earnings Date’: ‘Jul 28, 2020 – Aug 03, 2020’,
‘Ex-Dividend Date’: ‘May 08, 2020’,
‘Forward Dividend & Yield’: ‘3.28 (1.02%)’,
‘Market Cap’: ‘1.395T’,
‘Open’: 317.75,
‘PE Ratio (TTM)’: 25.29,
‘Previous Close’: 317.94,
‘Quote Price’: 321.8500061035156,
‘Volume’: 20185053.0}
Get the P/E ratio by:
quote_table[“PE Ratio (TTM)”]
25.29 - If you want to receive the dividends that you are expecting from a stock, then use the get_quote_table() function as part of Yahoo Finance Stock API quote_table = si.get_quote_table(“aapl”)quote_table[“Forward Dividend & Yield”] ‘3.28 (1.02%)’
-
If you want to download the trading data like historical open, high, low, close, and volume data sampled at regular intervals then use the get_data() method
si.get_data(“aapl”) - Among the Yahoo Finance API codes, the one to get the market cap is si.get_quote_table(“aapl”)[“Market Cap”] ‘1.39T’
-
The 52 week average volume can be retrieved from the following code
si.get_quote_table(“aapl”)[“Avg. Volume”]
si.get_quote_table(“aapl”)[“Volume”]
49012080.0
7274811.0 - To download the data from the income statement, you need to use get_income_statement() income_statement = si.get_income_statement(“aapl”)
-
To download the data from the balance sheet, you need to use get_balance_sheet()
balance_sheet = si.get_balance_sheet(“aapl”) - To download the data from the cash flow statement, you need to use get_cash_flow() cash_flow_statement = si.get_cash_flow(“aapl”)
- To download the options data, you need to use the code import yahoo_fin.options as ops
Let’s try to understand the different ways to access the Yahoo Finance Charts API or any other Yahoo Finance API.
- RapidAPI – It supports Python and helps you to pick from 15 different types of programming languages
- yfinance – open source library which is easy to set up and install $ pip install yfinance –upgrade –no-cache-dir
- Yahoo_fin – another open-source library widely used.
For more details please refer to Yahoo API documentation
Yahoo finance API documentation references
To know more you need to refer to Yahoo Finance API documentation. Here are some of the key links to refer to:
- For details on the OAuth model and protocol required for authorization and authentication, check out the link on the documentation. It provides a detailed guide on how to use OAuth along with the FAQs and Troubleshooting.
- For details on Yahoo App Publishing, refer to this link
- The Yahoo Finance API docs and reference resources are available at this location
- If you want to specifically refer to the Yahoo Finance API tutorial for Android, then refer to this link
- The Yahoo Finance Rest API documentation for iOS- reference documentation
- If you want to check out the video tutorials related to Yahoo Finance Chart API documentation, click here
- You can also go through the sample codes that will help in your app development.
Yahoo finance API example
Now let us focus on some Yahoo Finance API example, that will ease the development efforts and can serve as a ready reference while building an app. The following sub-sections will focus on certain critical technologies leveraged for building the integration. You can refer to the website for more details.
1. How to use Yahoo Finance Python API
The first step in how to use Yahoo Finance Python API is to import all the required yahoo finance API Python libraries.
The Yahoo Finance API Python example for importing libraries is
import pandas as pd
import yfinance as yf
The second step is to download the data from Python Yahoo Finance API, and the sample code for it is
df_yahoo = yf.download(‘FB’,
start=’2020-09-15′,
end=’2020-11-15′,
progress=False
You can also download multiple tickers, the Yahoo Finance API Python example code for it is
df_yahoo = yf.download([‘FB’,”AAPL”],
start=’2020-09-15′,
end=’2020-11-15′,
progress=False
2. How to use Yahoo Finance API Javascript
Here is a brief guide on how to use Yahoo Finance API Javascript.
If you want to make multiple requests then use the code
/v11/finance/quoteSummary/{symbol}
Next, let us check the sample code if you want to get the numbers using the price and summaryDetail modules.
var axios = require(“axios”).default;
var options = {
method: ‘GET’,
url: ‘https://rest.yahoofinanceapi.com/v11/finance/quoteSummary/AAPL’,
params: {modules: ‘defaultKeyStatistics,assetProfile’},
headers: {
‘x-API-key’: ‘YOUR-PERSONAL-API-KEY
}
};
axios.request(options).then(function (response) {
console.log(response.data);
}).catch(function (error) {
console.error(error);
});
3. How to use Yahoo Finance API Google sheets
To understand how to use Yahoo Finance API Goole sheets, we need to focus on
IMPORTXML, one of the best ways to import data into Google Sheets.
First you need to set the template using =IMPORTXML(url, xpath_query)
Get the URL by copying it and pasting on Google Sheets
Get the XPaths for the elements to be imported like market cap, price, volume, and earnings date.
Set up the template using the URL and XPaths
All the above elements are referred to as tickers.
Finally import the data from the companies as per the needs.
4. How to use Yahoo Finance API Excel
To use Yahoo Finance API Excel, there are three methodologies or ways:
- The easiest method is to use Coupler.io. There is an ETL tool available wherein Excel and the JSON API can be integrated. Alternatively, a request can be submitted to the data service of Coupler.io for consumption and setting up the integration with Yahoo Finance API.
- The next method is to use Power Query for extracting information from Yahoo Finance API. The Yahoo Finance CSV API documentation in https://developer.yahoo.com/api/ contains additional details
- One more method is to create a program using Python to extract the data.
5. How to use Yahoo Finance API JSON
Yahoo Finance API JSON has several modules which are leveraged for accessing various information.
- The assetProfile is a typical Yahoo finance API json example that helps in accessing information about the company specifically the assets
- recommendationTrend is another parameter that helps to understand the recommendations from analysts
- financialData is a vital parameter of the Yahoo Finance API module that can be used to find out the financial situation of a company
- To know about the company’s earnings, the earnings parameter is vital
- netSharePurchaseActivity is a parameter considered to be crucial for retrieving information about the net purchase activity of the company
6. How to use Yahoo Finance API Java
Yahoo Finance API Java is used through various methods by accepting a String [ ] of symbols for retrieving quotes and additional information about the stocks.
A Java Yahoo Finance API example is mentioned below where requests for essential quotes can be sent
get(String[] symbols)
Various other methods within yahoo finance java API help to extract dividend and statistics data. Separate requests can also be sent out for retrieving historical data. Even forex rates can be received by using the method getFx(String symbol)
7. How to use Yahoo Finance API C#
Yahoo Finance API C# examples for understanding its usage to extract pricing data, dividend data, volume data, average data, and ratio data
var yahooFinanceClient = new YahooFinance.YahooFinance();
var apple = yahooFinanceClient.RetrieveStock(“AAPL”);
Console.WriteLine($”Ask Price is {apple.PricingData.Ask}”);
Console.WriteLine($”Bid Price is {apple.PricingData.Bid}”);
Console.WriteLine($”Open Price is {apple.PricingData.Open}”);
Console.WriteLine($”Previous Close is {apple.PricingData.PreviousClose}”);
var yahooFinanceClient = new YahooFinance.YahooFinance();
var apple = yahooFinanceClient.RetrieveStock(“AAPL”);
Console.WriteLine($”Volume is {apple.VolumeData.CurrentVolume}”);
Console.WriteLine($”Ask Size is {apple.VolumeData.AskSize}”);
Console.WriteLine($”Bid Size is {apple.VolumeData.BidSize}”);
Console.WriteLine($”Last Trade Size is {apple.VolumeData.LastTradeSize}”);
8. How to use Yahoo Finance API Android Studio
To use Yahoo Finance API Android Studio app there is a console provided for development and establishing linkage between Android and Yahoo Finance API.
If you need the Android App to be continuously refreshed with the live data then you need to use the PHP mechanism. Whereas if you want the data to be refreshed at certain intervals, the server side code can be leveraged. Auto refresh or pull refresh methodology can be adopted to get the live feed into the Android App. There is also a provision for using AJAX but it is not advisable to use it since it introduces performance issues.
9. How to use R API for Yahoo Finance
To use R API for Yahoo Finance, here are the main steps:
Get access to the data by referencing the following sample code:
- install.packages(“quantmod”)
- library(quantmod)
Get data by referring to the example code
# Define the tickers so we formulate what we need
tickers <- c(“JPM”, “BAC”, “WFC”)
# Download stock data
stock_data <- new.env()
getSymbols(tickers, src = ‘yahoo’, env = stock_data, from = ‘2020–01–01’, to = Sys.Date())
Visualize data by leveraging the following sample code:
# Merge adjusted closing prices
jpm_adj_close <- data.frame(Date=index(jpm_data),
Ticker=“JPM”,
Adjusted=as.numeric(jpm_data$JPM.Adjusted))
bac_adj_close <- data.frame(Date=index(bac_data),
Ticker=“BAC”,
Adjusted=as.numeric(bac_data$BAC.Adjusted))
wfc_adj_close <- data.frame(Date=index(wfc_data),
Ticker=“WFC”,
Adjusted=as.numeric(wfc_data$WFC.Adjusted))
combined_adj_close <- rbind(jpm_adj_close, bac_adj_close, wfc_adj_close)
Also read: How to use Instagram API – a step-by-step integration guide and full documentation list
Yahoo Finance API pricing
The Yahoo Finance API free basic plan is used for accessing 500 requests in a month and the rate limit is 5 requests per second. The Yahoo Finance API price for the Pro plan is USD 10 per month where you can access 10,000 requests in a month and the rate limit is the same as that of basic Yahoo Finance API pricing which is 5 in number per second.
Most used Yahoo Finance APIs
Let us now discuss the most used Finance APIs. These APIs will help in the development activities and also the purpose of using them. In the sub-sections, we will focus on a brief overview of each of the key Yahoo Finance APIs.
Yahoo Finance API historical data
Yahoo Finance API historical data is used to retrieve historical data for various financial markets and products.
Yahoo Finance news API
Yahoo Finance news API is used to find out the news about stocks, mutual funds, options, and ETFs.
Yahoo Finance API symbol list
Yahoo Finance API symbol list is used to find out the ticker symbols for stocks, mutual funds, options, ETFs, futures, and indices.
Yahoo Finance API exchange rate
Yahoo Finance API exchange rate is used to find out the conversion rate between two currencies and also the historical exchange rates.
Yahoo Finance query API
Yahoo Finance query API is used to extract information related to quotes, stocks, financial summaries, etc. which are searched similarly on the website.
Yahoo Finance API options
Yahoo Finance API Options is used to retrieve information and analysis related to options in a financial market or portfolio.
Yahoo Finance market data API
Yahoo Finance Market Data API is used to receive historical and real-time data related to the financial markets.
Yahoo Finance crypto API
Yahoo Finance Crypto API helps to access the list of top cryptocurrencies and their prices.
Yahoo Finance key statistics API
Yahoo Finance key statistics API is used to extract the key statistics of a company like the fiscal year financial statistics, valuation metrics, share statistics, and many more.
Yahoo Finance API TAGS
Yahoo Finance API TAGS helps to extract information related to the stock, quotes, news, and history of TAGS.
Yahoo Finance feed API
Yahoo Finance Feed API is used to retrieve the latest updates related to business and financial news.
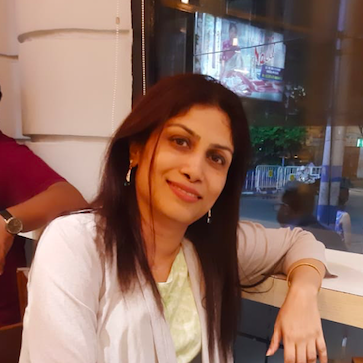
Kuntala is a versatile writer with a focus on diverse areas around work, productivity, collaboration at work, hiring, management, HR, and training. Her background of past experience in technology and consulting helps in molding razor-sharp insights into the research and user-focused content she creates. Professionally she is an IT consultant in a sales role and also a writer of short stories and poems, travel blogger, and fashion influencer.
Leave a Reply